Welcome to the world of Python game development! In this project, we’ll embark on a journey to create a classic game of Tic-Tac-Toe using Python. Tic-Tac-Toe is a fantastic choice for beginners because it not only introduces you to Python programming but also allows you to explore fundamental game development concepts.
Jump to:
- Set-Up-Your-Development-Environment
- Design the Game Board
- Display the Game Board
- Implement Game Logic
- Coding the Game
- Game Instructions
Project Goal: Our goal is to build a two-player Tic-Tac-Toe game from scratch. You’ll learn how to create the game’s logic, manage player input, and even implement a simple graphical user interface (GUI) if you’re up for an extra challenge. Whether you’re a newbie to Python or looking to strengthen your coding skills, this project is an excellent way to do it while having fun.
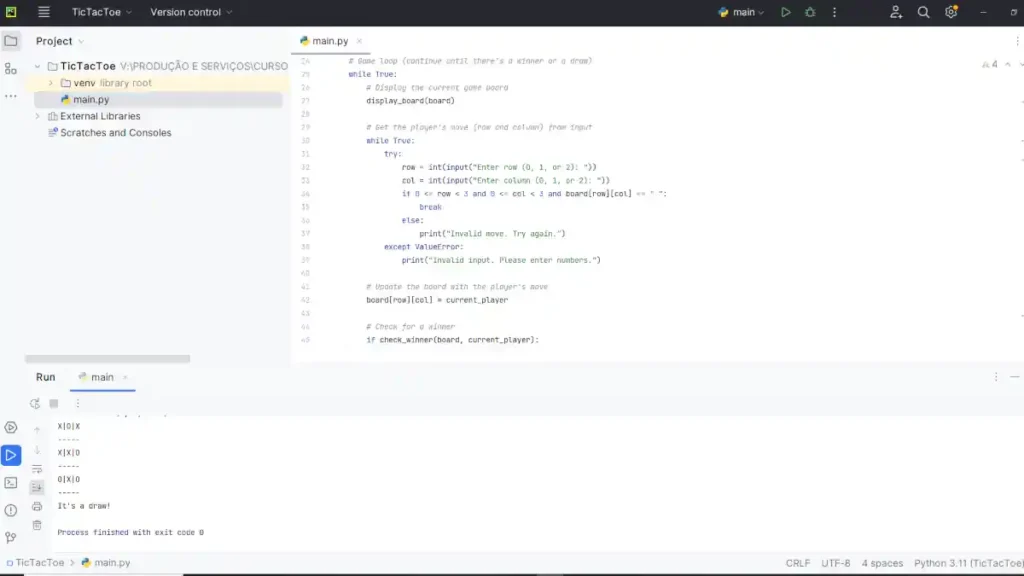
Set Up Your Development Environment
Before we dive into coding our Tic-Tac-Toe game, we need to set up our development environment. This ensures that you have all the necessary tools and software in place to make the coding process smooth.
Step 1: Install Python
If you haven’t already installed Python, it’s time to do so. Python is the programming language we’ll use to create our game. Follow these steps to install Python:
- Visit the official Python website at python.org.
- Download the latest version of Python for your operating system (Windows, macOS, or Linux).
- Run the installer and follow the installation instructions.
- Check the box that says “Add Python to PATH” during installation. This makes it easier to run Python from the command line.
To check if Python was installed correctly, open your command prompt (Windows) or terminal (macOS and Linux) and type:
python --version
You should see the installed Python version displayed.
Step 2: Choose a Code Editor or IDE
Next, you’ll need a code editor or Integrated Development Environment (IDE) to write your Python code. There are several options available, and you can choose the one that suits you best. Here are a few popular choices:
- Visual Studio Code (VS Code): A highly customizable and feature-rich code editor with excellent Python support. Download it from code.visualstudio.com.
- PyCharm: A Python-specific IDE developed by JetBrains. It offers many useful features for Python development. You can download the community edition for free from jetbrains.com/pycharm.
- IDLE: IDLE is Python’s built-in IDE, and it’s a straightforward option for beginners. It comes bundled with Python when you install it.
Choose the code editor or IDE that you’re most comfortable with or explore multiple options to see which one you prefer. Once you’ve installed your chosen development environment, you’re ready to start building your Tic-Tac-Toe game!
Design the Game Board
In the world of programming, it’s essential to plan and structure your data before you start building your game. For our Tic-Tac-Toe game, we’ll begin by designing the game board.
Step 1: Create a 3×3 Grid
To represent the Tic-Tac-Toe game board, we’ll create a 3×3 grid. Each cell in this grid will correspond to a position where X or O can be placed. There are various ways to implement this grid, but a common choice is to use a list of lists.
Here’s a simple example of how you can create the initial game board using a list of lists in Python:
# Initialize an empty 3x3 grid for Tic-Tac-Toe
board = [
[" ", " ", " "],
[" ", " ", " "],
[" ", " ", " "]
]
In this representation:
- Each element in the outer list represents a row on the game board.
- Each element in the inner lists represents a cell within that row.
- We use spaces (” “) to indicate empty cells.
Feel free to choose other data structures or representations if you prefer. The key is to have a clear way to track the state of the game board and update it as players make their moves.
Now that we have our initial game board design, we can move on to displaying it and implementing the game logic.
Display the Game Board
In this section, we’ll work on displaying the Tic-Tac-Toe game board. How you choose to display it depends on whether you want a simple console-based interface or a more user-friendly graphical interface. We’ll cover both options.
Option 1: Console-Based Display
To display the game board in the console, you can create a function that prints the current state of the board. Here’s an example of how you can do it:
def display_board(board):
for row in board:
print("|".join(row))
print("-" * 5)
You can call this display_board
function to show the game board to players after each move.
Option 2: Graphical Interface with Tkinter (Optional)
If you want to create a graphical user interface (GUI) for a more user-friendly experience, you can use the Tkinter library, which comes with Python. Building a GUI involves more code and complexity, but it can make the game more enjoyable to play.
Here’s a basic example of how to create a Tic-Tac-Toe GUI using Tkinter:
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Tic-Tac-Toe")
# Create buttons for each cell in the game board
buttons = [[tk.Button(root, text=" ", height=2, width=5) for _ in range(3)] for _ in range(3)]
# Place the buttons in a grid layout
for i in range(3):
for j in range(3):
buttons[i][j].grid(row=i, column=j)
# Run the GUI main loop
root.mainloop()
This code creates a simple GUI with buttons for each cell in the game board. You can customize and enhance the GUI as desired.
Implement Game Logic
Now, let’s move on to implementing the game logic. In Tic-Tac-Toe, we need to:
- Track whose turn it is (X or O).
- Allow players to make moves.
- Check for winning conditions (a player gets three in a row) or a draw.
Here’s a high-level outline of how you can structure the game logic:
# Initialize the game board and player's turn
board = [[" " for _ in range(3)] for _ in range(3)]
current_player = "X"
# Game loop (continue until there's a winner or a draw)
while True:
# Display the current game board
display_board(board)
# Get the player's move (row and column) from input or GUI interaction
# Update the board with the player's move
# Check for a winner (three in a row) or a draw
# If there's a winner, display the result and exit the loop
# If it's a draw, display a draw message and exit the loop
# Switch to the other player's turn (X -> O or O -> X)
Implementing these steps will complete the core game logic. You’ll need to handle player input, check for winning conditions, and ensure the game continues until there’s a winner or a draw. This forms the heart of your Python Tic-Tac-Toe game!
Coding the Game
Here’s a Python Tic-Tac-Toe game code that includes the game board display and logic. This code is for a console-based game. To run it, simply copy and paste it into a Python environment or a script file and execute it.
def display_board(board):
for row in board:
print("|".join(row))
print("-" * 5)
def check_winner(board, player):
# Check rows, columns, and diagonals for a win
for i in range(3):
if all(board[i][j] == player for j in range(3)) or all(board[j][i] == player for j in range(3)):
return True
if all(board[i][i] == player for i in range(3)) or all(board[i][2 - i] == player for i in range(3)):
return True
return False
def is_draw(board):
# Check if the game is a draw (all cells are filled)
return all(board[i][j] != " " for i in range(3) for j in range(3))
def main():
# Initialize the game board and player's turn
board = [[" " for _ in range(3)] for _ in range(3)]
current_player = "X"
# Game loop (continue until there's a winner or a draw)
while True:
# Display the current game board
display_board(board)
# Get the player's move (row and column) from input
while True:
try:
row = int(input("Enter row (0, 1, or 2): "))
col = int(input("Enter column (0, 1, or 2): "))
if 0 <= row < 3 and 0 <= col < 3 and board[row][col] == " ":
break
else:
print("Invalid move. Try again.")
except ValueError:
print("Invalid input. Please enter numbers.")
# Update the board with the player's move
board[row][col] = current_player
# Check for a winner
if check_winner(board, current_player):
display_board(board)
print(f"Player {current_player} wins!")
break
# Check for a draw
if is_draw(board):
display_board(board)
print("It's a draw!")
break
# Switch to the other player's turn (X -> O or O -> X)
current_player = "O" if current_player == "X" else "X"
if __name__ == "__main__":
main()
Game Instructions
Welcome to the world of Python game development! In this project, we’ve crafted a classic game of Tic-Tac-Toe using Python. Tic-Tac-Toe is not just a childhood favorite; it’s also a fantastic way to delve into Python programming and explore the foundations of game development.
Project Overview:
This Python script will allow you to play the timeless game of Tic-Tac-Toe right in your console. The game is designed for two players, “X” and “O,” who take turns marking their moves on a 3×3 grid. The first player to get three in a row, horizontally, vertically, or diagonally, wins the game. If all cells on the board are filled without a winner, the game ends in a draw.
How to Play:
- Players take turns specifying the row and column where they want to place their symbol.
- The game checks for winning conditions or a draw after each move.
- The player who achieves three in a row first is declared the winner.
- If all cells are filled without a winner, the game ends in a draw.
Now, let’s dive into the Python code and see how we’ve implemented this timeless game. Feel free to run the code, challenge your friends, and explore the inner workings of this Python-powered Tic-Tac-Toe game!