Data Access Objects (DAO) play a pivotal role in the architecture of software applications, providing an abstraction layer over database access. By isolating the application/business layer from the data access layer, DAOs ensure that the database interactions in an application are robust, flexible, and easy to manage.
This concept, crucial for students and professionals in computer science and software engineering, allows for cleaner code and easier maintenance. In this article, we will delve into the workings of DAOs, their importance, and their implementation, along with a historical view of related technologies, such as those once promoted by Microsoft but now deprecated.
Index:
- What is a Data Access Object (DAO)?
- Understanding DAO Architecture
- Implementing DAOs: Best Practices
- DAOs and Database Interactions
- DAO Frameworks and Technologies
- The Role of DAOs in Modern Software Development
- Deprecated Microsoft Technologies for Database Access
- References
1. What is a Data Access Object (DAO)?
Definition and Importance of DAO in Software Development
A Data Access Object (DAO) is a design pattern that provides an abstract interface to a database or other persistence mechanism. By mapping application calls to the persistence layer, DAOs provide specific data operations without exposing details of the database. This abstraction is crucial for minimizing dependencies between the application’s core logic and the underlying database management systems.
The importance of DAOs in software development stems from their role in enforcing separation of concerns. By decoupling the data access code from the business logic, DAOs promote a cleaner, more scalable, and maintainable codebase. This separation allows developers to modify the database access strategy or switch databases altogether without impacting the business logic layer.
Overview of the DAO Pattern and Its Components
The DAO pattern typically involves several key components:
- DAO Interface: This defines the standard operations to be performed on a model object(s).
- DAO Implementation: This component implements the DAO interface and contains the logic required to handle database operations.
- Data Transfer Object (DTO): Sometimes also known as Value Objects, these are simple objects that do not contain any business logic but rather carry data between processes.
- Database: The actual database on which operations are performed.
The structure of DAOs ensures that all database operations are centralized in one part of the application, thus maintaining organization and readability.
2. Understanding DAO Architecture
Detailed Explanation of the DAO Architecture
DAO architecture typically consists of multiple layers that abstract the processes involved in managing database transactions. At its core, the DAO layer sits between the business service layer and the database, acting as a mediator that communicates data requests and updates:
- Service Layer: This layer interacts with the DAO, requesting data operations without needing knowledge of underlying database specifics.
- DAO Layer: It translates those requests into concrete database queries or transactions. This layer handles all the complexities of the database operations.
- Persistence Layer: Sometimes integrated with the DAO layer, it configures how data is persisted and retrieved, often using an ORM tool to map objects to database tables directly.
How DAOs Separate Data Access Logic from Business Logic
Separation of data access logic from business logic is achieved by isolating responsibilities. The business logic layer focuses on computational logic and decision making, involving rules, calculations, and conditions. In contrast, the data access logic layer is solely concerned with data retrieval and manipulation. This separation is vital for reducing dependencies, thereby facilitating easier updates and maintenance. Changes in business rules do not affect data handling mechanisms, and vice versa, ensuring that enhancements or bug fixes in one area do not ripple through to others.
Benefits of Using the DAO Pattern in Application Development
Using the DAO pattern in application development offers several benefits:
- Enhanced Maintainability: Changes in database structure or the switch to a different database provider impact only the DAO implementation, leaving business logic unaffected.
- Improved Testability: With a clear separation of concerns, it becomes easier to write unit tests for both business logic and data access code independently.
- Flexibility: Developers can work on business logic and database management simultaneously with less risk of conflicts, as interfaces define clear contracts.
- Scalability: As business requirements evolve, the application can scale more smoothly due to the decoupled nature of its architecture. For example, introducing new forms of data storage or data sources requires changes only in the DAO layer.
Overall, the DAO pattern is instrumental in building robust, scalable applications that can adapt to changes in business strategies or technology environments efficiently.
3. Implementing DAOs: Best Practices
Step-by-Step Guide on Implementing DAOs in Various Programming Languages
Implementing the Data Access Object (DAO) pattern can vary significantly across different programming languages and frameworks. Here, we’ll outline a basic approach that can be adapted to fit specific environments:
- Define the DAO Interface: Start by defining an interface that lists all the operations you expect to perform on the database, such as
add
,delete
,update
, andfind
. - Create Data Transfer Objects (DTOs): Define your DTOs which will carry data between the DAO and other parts of your application. These should be simple objects, ideally without business logic.
- Implement the DAO Interface: Write a class that implements the defined interface. This class will handle the database operations required by the interface methods.
- Configure Database Connection: Establish a connection to your database. This could be done within the DAO implementation or externally through a database connection pool for efficiency.
- Integrate with the Business Logic: Use the DAO in your business logic layer by calling the methods defined in your DAO interface.
Examples of DAO Implementation
Here is a detailed example of a simple DAO implementation in Java using JDBC:
public interface CustomerDAO {
List<Customer> findAll();
Customer findById(int id);
void create(Customer customer);
void update(Customer customer);
void delete(int id);
}
public class CustomerDAOImpl implements CustomerDAO {
private Connection connection;
public CustomerDAOImpl(Connection connection) {
this.connection = connection;
}
@Override
public List<Customer> findAll() {
List<Customer> customers = new ArrayList<>();
try {
Statement statement = connection.createStatement();
ResultSet rs = statement.executeQuery("SELECT * FROM customers");
while (rs.next()) {
Customer customer = new Customer(rs.getInt("id"), rs.getString("name"), rs.getString("email"));
customers.add(customer);
}
} catch (SQLException e) {
e.printStackTrace();
}
return customers;
}
@Override
public Customer findById(int id) {
Customer customer = null;
try {
PreparedStatement statement = connection.prepareStatement("SELECT * FROM customers WHERE id = ?");
statement.setInt(1, id);
ResultSet rs = statement.executeQuery();
if (rs.next()) {
customer = new Customer(rs.getInt("id"), rs.getString("name"), rs.getString("email"));
}
} catch (SQLException e) {
e.printStackTrace();
}
return customer;
}
@Override
public void create(Customer customer) {
try {
PreparedStatement statement = connection.prepareStatement("INSERT INTO customers (name, email) VALUES (?, ?)");
statement.setString(1, customer.getName());
statement.setString(2, customer.getEmail());
statement.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
}
@Override
public void update(Customer customer) {
try {
PreparedStatement statement = connection.prepareStatement("UPDATE customers SET name = ?, email = ? WHERE id = ?");
statement.setString(1, customer.getName());
statement.setString(2, customer.getEmail());
statement.setInt(3, customer.getId());
statement.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
}
@Override
public void delete(int id) {
try {
PreparedStatement statement = connection.prepareStatement("DELETE FROM customers WHERE id = ?");
statement.setInt(1, id);
statement.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
Common Pitfalls and How to Avoid Them
- Hardcoding SQL Queries: Hardcoding can lead to inflexible code and potential SQL injection vulnerabilities. Use prepared statements or ORM frameworks to mitigate these risks.
- Poor Error Handling: Robust error handling is crucial. Ensure that your DAOs gracefully handle SQL exceptions and return meaningful errors to the application.
- Resource Leaks: Always ensure that database connections, statements, and result sets are properly closed after use to avoid leaking resources.
4. DAOs and Database Interactions
How DAOs Interact with Databases
DAOs act as an intermediary between the application and the database. They use the database connection to execute SQL queries or use an ORM framework to manage database interactions. This encapsulation of data access allows for cleaner and more manageable code.
CRUD Operations Within DAOs
CRUD operations—Create, Read, Update, Delete—are the backbone of database interactions in DAOs:
- Create: Insert new records into the database.
- Read: Retrieve data from the database. This can be a single item or a list of items based on some criteria.
- Update: Modify existing records in the database.
- Delete: Remove records from the database.
Managing Database Connections and Transactions Through DAOs
- Connection Management: It’s often efficient to use a connection pool to manage database connections. This minimizes the overhead of opening
5. DAO Frameworks and Technologies
Overview of Popular Frameworks and Technologies That Support the DAO Pattern
Various frameworks and libraries across programming languages have been developed to simplify the implementation of the DAO pattern. Here are some of the most notable ones:
- Java: The Spring Framework’s Data Access Object support and Java Persistence API (JPA) are widely used. Spring JDBC Template simplifies JDBC operations and error handling.
- .NET: Entity Framework provides a robust ORM framework that abstracts database interactions, allowing developers to work with data as strongly typed objects.
- Python: SQLAlchemy and Django ORM are popular choices, offering powerful ORM capabilities to abstract database operations.
- JavaScript/Node.js: Mongoose for MongoDB and Sequelize for SQL databases are common ORMs that manage data interactions in a structured way.
Comparative Analysis of These Frameworks
- Spring Framework (Java): Offers comprehensive data management capabilities, including transaction management, JDBC abstraction, and exception handling. Best suited for applications that require robustness and mature integration capabilities.
- Entity Framework (.NET): Provides a feature-rich, automated ORM capability with strong Microsoft SQL Server integration. Ideal for applications deeply integrated into the Microsoft ecosystem.
- SQLAlchemy (Python): Known for its flexibility and the power to handle both high-level ORM and low-level SQL, SQLAlchemy is great for applications that need fine-grained control over their database operations.
- Mongoose (Node.js): Streamlines interaction with MongoDB through a simple schema-based solution that manages relationships and validation effectively. Best for applications that use MongoDB as their database.
How to Choose the Right Framework for Your Project
Selecting the right DAO framework involves considering several factors:
- Project Requirements: Understand the database operations your project requires. Look for frameworks that provide specific features that meet these needs.
- Database Type: Choose a framework that best supports the type of database you are using (SQL vs. NoSQL).
- Community and Support: Opt for frameworks with a large community and extensive documentation. This can help with troubleshooting and adopting best practices.
- Performance: Evaluate the performance implications of each framework. Some frameworks may introduce overhead that could impact the application’s scalability.
- Learning Curve: Consider the ease of learning and using the framework, especially if project timelines are tight.
6. The Role of DAOs in Modern Software Development
The Relevance of DAOs in Today’s Software Architecture
In modern software architecture, DAOs continue to be highly relevant as they facilitate clean separation of concerns, which is a principle central to good architectural design. They enable the modularization of data access logic, making systems easier to maintain, test, and scale. As applications grow and evolve, having a well-defined DAO layer allows teams to adapt more quickly to changes in business requirements or underlying database technologies.
Case Studies Showing the Effective Use of DAOs in Real-World Applications
- E-commerce Platform: An e-commerce company implemented DAOs to handle the diverse and complex data interactions required by its online store. The DAO layer managed interactions with a SQL database for transactional data and a NoSQL database for user behavior analytics. This approach allowed them to scale their operations efficiently during high-traffic events like sales and holidays.
- Healthcare Application: A healthcare application used DAOs to ensure compliance with regulations like HIPAA, which requires strict data handling procedures. The DAO layer abstracted all data access into a secure module, making it easier to implement auditing and logging mechanisms that are critical for compliance.
- Banking System: A large bank integrated DAOs to decouple business logic from their data storage solutions. This separation allowed them to switch underlying databases from a legacy system to a more modern solution with minimal changes to the business logic, thereby reducing risk and downtime during the transition.
In each of these cases, the DAO architecture not only supported essential functionality but also enhanced the application’s ability to adapt to new challenges and requirements, showcasing the enduring value of DAOs in software development.
7. Deprecated Microsoft Technologies for Database Access
Historical Overview of Microsoft’s Data Access Objects (MS DAO) Technology
Microsoft’s Data Access Objects (DAO) technology was introduced in the early 1990s as part of the Microsoft Jet Database Engine, which powered databases like Microsoft Access. DAO was designed to allow Windows applications to access and manipulate data from a database through a series of programmable interfaces. During the mid to late 90s, DAO became a popular choice for desktop and small-scale applications, primarily because it was directly integrated into Microsoft Access and provided an easy way for developers to interface with local databases.
Reasons Behind Its Deprecation and Transition to Newer Technologies
Despite its initial popularity, DAO began to show limitations as enterprise applications grew in complexity and scale. Its dependence on the Jet Engine, which was not well-suited for high concurrency or large datasets, and its lack of support for features like transaction handling or connection pooling, made it less ideal for more robust applications. As web-based applications gained prominence, the need for more scalable and flexible data access solutions became apparent.
In response, Microsoft introduced newer technologies such as ActiveX Data Objects (ADO) and later ADO.NET, which were designed to address the shortcomings of DAO and to better meet the needs of modern applications, including support for disconnected data models and better integration with SQL Server. These newer models provided more flexibility, supported a wider range of data sources, and were better aligned with evolving developer practices and application scenarios.
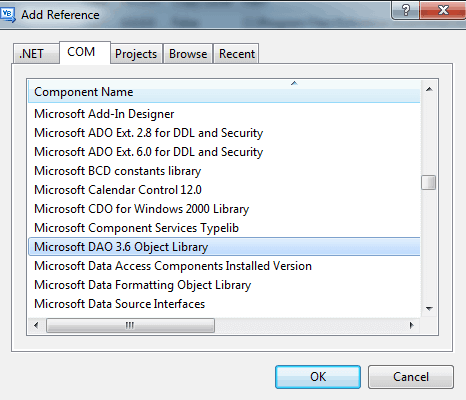
Comparison with Successors and Contemporaries Like ADO.NET
Compared to DAO, ADO.NET offers several advantages:
- Disconnected Model: ADO.NET is designed around a disconnected data model, meaning that data can be manipulated without a constant connection to the database, reducing the load on the database server and improving application performance.
- Multi-Database Support: While DAO was tightly coupled with Microsoft’s Jet Engine, ADO.NET supports multiple database platforms and is part of the broader .NET framework, making it suitable for a variety of development environments.
- Scalability and Performance: ADO.NET provides better scalability and performance for large applications, crucial for enterprise environments.
- Richer Set of Features: ADO.NET includes features such as XML integration, batch updates, and a more robust mechanism for error handling, which are essential for modern applications.
8. References
- “Programming Microsoft ADO.NET 2.0 Core Reference” by David Sceppa. This book offers insights into ADO.NET architecture and its core components, ideal for understanding the evolution from DAO.
- “Access Data Analysis Cookbook” by Ken Bluttman, Wayne S. Freeze. Although focused on Access, this book provides historical context on how DAO was used in Access databases.
- RFC 2549 – IP over Avian Carriers with Quality of Service: Although not directly related to DAO, understanding different transport protocols humorously highlights the adaptability needed in data handling technologies.
- “Expert One-on-One Visual Basic 2005 Database Programming” by Rod Stephens. This book gives practical examples and further discussions on moving from DAO to more modern frameworks in the context of Visual Basic programming.