Welcome to the intriguing world of MAPI, the Messaging Application Programming Interface, a cornerstone in the landscape of email and messaging systems. MAPI is more than just a protocol; it’s a bridge that connects messaging clients like Microsoft Outlook to a range of mail servers, enabling seamless communication and data exchange. In this comprehensive guide, we will unravel the complexities of MAPI, exploring its structure, functionality, and pivotal role in the world of electronic messaging. From basic concepts to advanced implementation, this article promises to equip you with an in-depth understanding of MAPI and its applications in real-world scenarios.
Table of Contents:
- What is MAPI?
- Understanding MAPI Architecture
- MAPI in Microsoft Outlook
- Developing with Messaging Application Programming Interface
- MAPI Integration and Configuration
- Security Aspects of MAPI
- MAPI vs. Other Messaging APIs
- Conclusion
- References
1. What is MAPI?
Messaging Application Programming Interface, also known as MAPI, is a Microsoft technology that allows developers to use the Microsoft Windows messaging subsystem for writing messaging applications. It provides a rich set of functions for managing emails, appointments, tasks, and contacts. Unlike simpler mail protocols such as IMAP or SMTP, MAPI offers deeper integration with mail servers, enabling features like calendar management, complex queries, and full synchronization of mail stores.
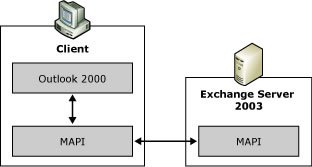
History and Evolution of MAPI
MAPI’s journey began in the early 1990s as part of Microsoft’s effort to enhance the capabilities of email clients. Originally designed to work with Microsoft Mail, MAPI evolved alongside Microsoft’s email and collaboration offerings, becoming integral to the functioning of Microsoft Exchange Server and Outlook. Over the years, MAPI has undergone several updates, each enhancing its functionality and integration capabilities. The evolution of MAPI mirrors the growing complexity and sophistication of email as a tool for personal and business communication.
2. Understanding MAPI Architecture
Core Components of MAPI
At its core, MAPI consists of a set of interfaces, properties, operations, and objects that provide a systematic way for applications to interact with the messaging system. The key components include the Client Interface Layer, the MAPI Layer (MAPI subsystem), and the Service Provider Interface Layer. These elements work together to facilitate complex messaging functions beyond basic send and receive capabilities.
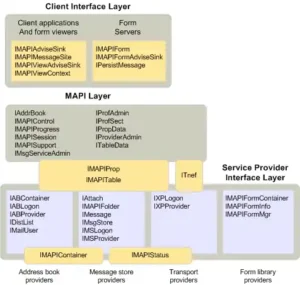
How MAPI Communicates with Mail Servers
MAPI communicates with mail servers using a client-server model. When an email client like Outlook uses MAPI, it calls on MAPI client libraries to interact with the MAPI subsystem. This subsystem then communicates with the appropriate service providers – such as message stores or address books – which in turn interact with the mail server to perform the requested operations. This layered communication allows MAPI to support sophisticated features like notifications, rules processing, and rich content handling.
MAPI and Microsoft Exchange Server
The relationship between MAPI and Microsoft Exchange Server is particularly significant. Exchange Server uses MAPI extensively for internal operations and exposes MAPI for external communication with clients. This deep integration allows for efficient synchronization of data, real-time updates, and extensive collaboration features that are characteristic of Exchange environments.
3. MAPI in Microsoft Outlook
Role of MAPI in Outlook
MAPI plays a pivotal role in Microsoft Outlook, acting as the underlying framework that facilitates communication between Outlook and Microsoft Exchange Server. It’s the driving force that enables Outlook to perform complex tasks beyond simple email sending and receiving. Through MAPI, Outlook gains the ability to manage calendars, tasks, contacts, and more, providing a comprehensive and integrated user experience.
MAPI Features and Functionalities in Outlook
The features and functionalities that MAPI brings to Outlook are extensive. These include:
- Rich Email Interaction: MAPI allows Outlook to handle rich-text emails, including formatted text, embedded images, and attachments.
- Calendar Management: MAPI enables full calendar functionalities, such as creating meetings, sending invites, and syncing calendars across devices.
- Task and Contact Management: Tasks and contacts are seamlessly managed and synchronized, thanks to MAPI’s deep integration.
- Notification Services: MAPI provides real-time notifications for new emails, calendar alerts, and task reminders.
4. Developing with Messaging Application Programming Interface
Setting Up the Development Environment
To start developing with MAPI, you’ll need:
- Microsoft Outlook: The application itself, as it includes the necessary MAPI libraries.
- Windows SDK: This provides additional tools and headers for MAPI development.
- A Development IDE: Like Visual Studio, for writing and testing your code.
Basic MAPI Programming Concepts
When delving into MAPI programming, some fundamental concepts to grasp include:
- MAPI Sessions: Understanding how to establish and terminate sessions with the messaging system.
- Messaging Objects: Learning to work with various MAPI objects like messages, folders, and stores.
- Properties and Interfaces: Getting familiar with MAPI’s property and interface system, which is key to manipulating messaging data.
Advanced MAPI Development Techniques
For more advanced development:
- Extended MAPI: Explore deeper functionalities offered by extended MAPI for complex tasks.
- Asynchronous Operations: Implement asynchronous processes for efficiency and enhanced user experience.
- Error Handling and Debugging: Develop robust error handling strategies and debugging techniques for reliable application performance.
Example: Reading Emails from Outlook Using MAPI
Setting Up the Development Environment
- Install Microsoft Outlook: Ensure that Microsoft Outlook is installed on your development machine, as it includes the necessary MAPI libraries.
- Install Windows SDK: The Windows Software Development Kit (SDK) provides additional headers and libraries for MAPI development.
- Choose a Development IDE: Use an IDE like Visual Studio for writing and testing your code. Set up a new project in the IDE for your MAPI application.
Accessing MAPI
- Initialize MAPI: Before any MAPI functions can be used, initialize the MAPI subsystem. This is typically done using the
MAPIInitialize
function.
MAPIINIT_0 MAPIInit = { 0, MAPI_MULTITHREAD_NOTIFICATIONS };
HRESULT hr = MAPIInitialize(&MAPIInit);
- Log on to a MAPI Session: Create a new MAPI session using
MAPILogonEx
, which allows you to log on to the messaging system.
LPMAPISESSION pSession = NULL;
hr = MAPILogonEx(0, NULL, NULL, MAPI_USE_DEFAULT, &pSession);
Retrieving Email Data
- Open the Default Message Store: Access the default message store where emails are stored.
LPMDB pMDB = NULL;
hr = pSession->OpenMsgStore(0, 0, NULL, NULL, MDB_NO_DIALOG | MDB_NO_MAIL, &pMDB);
- Access the Inbox Folder: Once you have access to the message store, open the Inbox folder.
LPMAPIFOLDER pInbox = NULL;
hr = pMDB->OpenEntry(0, NULL, NULL, MAPI_BEST_ACCESS, &pInbox);
- Enumerate Messages in the Inbox: Loop through the messages in the Inbox and read their properties, such as subject and sender.
LPMAPITABLE pContentsTable = NULL;
hr = pInbox->GetContentsTable(0, &pContentsTable);
// Set columns, restrict and loop through messages to read properties
- Cleanup: Release all MAPI objects and uninitialize MAPI.
pContentsTable->Release();
pInbox->Release();
pMDB->Release();
pSession->Release();
MAPIUninitialize();
This is a basic example of using MAPI to access and read emails from an Outlook mailbox. It involves initializing MAPI, logging onto a session, accessing the message store, and then iterating through the Inbox to read email properties.
5. MAPI Integration and Configuration
Integrating MAPI with Different Mail Servers
Integrating MAPI with various mail servers, including but not limited to Microsoft Exchange, requires understanding the specific protocols and configurations each server uses. For non-Exchange servers, this often involves setting up MAPI to work with IMAP or POP3 protocols. The key is ensuring that MAPI can correctly translate and synchronize data across different server types, maintaining consistent functionality for end-users.
Configuration and Troubleshooting
Configuring MAPI correctly is crucial for seamless operation. This involves:
- Setting Up Profiles and Services: Ensuring that user profiles are correctly configured to connect with the desired mail servers.
- Addressing Connectivity Issues: Regularly checking and troubleshooting connectivity issues, such as authentication errors or server unavailability.
- Updating and Patching: Keeping the MAPI client and server components updated to the latest versions to prevent compatibility issues.
6. Security Aspects of MAPI
Security Features in MAPI
MAPI incorporates several security features to protect data integrity and confidentiality:
- Encryption: MAPI supports encryption to secure data during transmission.
- Authentication Protocols: It utilizes robust authentication protocols to verify user identities and prevent unauthorized access.
- Access Control: MAPI allows for detailed access control settings, ensuring that users can only access the data and functions relevant to them.
Best Practices for Secure MAPI Implementation
To maximize MAPI’s security, adhere to these best practices:
- Regular Updates: Keep all software involved in MAPI communication up-to-date to protect against vulnerabilities.
- Strong Authentication Measures: Implement strong passwords and, where possible, multi-factor authentication.
- Monitoring and Auditing: Regularly monitor and audit MAPI interactions to detect and respond to suspicious activities promptly.
7. MAPI vs. Other Messaging APIs
Comparison with IMAP, POP3, and SMTP
When contrasting MAPI with other common messaging APIs like IMAP, POP3, and SMTP, several key differences emerge:
- Complexity and Capability: MAPI is more complex than IMAP, POP3, and SMTP but offers a wider range of features, including comprehensive mail store access, calendar management, and advanced query capabilities.
- Server Interaction: IMAP and POP3 are primarily focused on email retrieval from the server, with SMTP being used for sending emails. MAPI, on the other hand, provides a more integrated and interactive server-client relationship.
- Usage Scenarios: While IMAP, POP3, and SMTP are sufficient for basic email sending and receiving, MAPI is tailored for scenarios requiring deeper integration with the mail server and extensive user interaction.
Choosing the Right API for Your Needs
Selecting the appropriate API depends on specific needs:
- Simplicity vs. Functionality: For basic email functionalities, IMAP, POP3, and SMTP are suitable. However, for applications needing complex interactions like Outlook, MAPI is the go-to choice.
- Integration Requirements: If deep integration with Microsoft Exchange Server is required, MAPI is the preferred option.
8. Conclusion
MAPI stands out as a powerful tool in the realm of messaging APIs, offering advanced functionalities and deep integration capabilities, particularly within Microsoft’s ecosystem. Understanding its nuances, comparisons with other APIs, and real-world applications equips professionals with the knowledge to make informed decisions regarding their messaging infrastructure.
9. References
For further exploration into MAPI and related technologies, consider these resources:
- “Programming Applications for Microsoft Office Outlook 2007” by Randy Byrne and Ryan Gregg: Detailed insights into MAPI’s role in Outlook.
- “Microsoft Exchange Server 2016 PowerShell Cookbook” by Jonas Andersson: For advanced MAPI scripting and automation.
- “Outlook MAPI Reference Overview” by Microsoft Learn.
- Online Resources: Microsoft’s documentation, developer forums, and knowledge bases for the latest on MAPI development and best practices.