In the expansive realm of computer programming, few methodologies have garnered as much attention, adoption, and acclaim as the object-oriented approach. From its early days of conception to its modern-day significance, object-oriented languages have reshaped the way developers envision, design, and implement software solutions. For budding computer science enthusiasts and seasoned developers alike, understanding the essence of object-oriented languages is paramount. It’s not just a matter of mastering a particular language; it’s about embracing a philosophy, a distinct way of thinking about problems and their solutions.
In this article:
- What is an Object-Oriented Language?
- Historical Overview of OOL: Tracing back the roots of object-oriented design
- Core Pillars of OOP: An in-depth look at Encapsulation, Inheritance, Polymorphism, and Abstraction
- Comparison with Procedural Languages: Highlighting the distinctions between OOP and procedural programming
- Real-world applications of OOP
- Main Object-Oriented Languages
- Code Examples: Object Creation, Properties, and Methods in Python
- Essential Reading: Comprehensive Books on Object-Oriented Programming
- Conclusion
But what is it about object-oriented programming (OOP) that makes it stand out from other methodologies? What makes it a go-to choice for many when developing complex software systems? Join us on this enlightening journey as we dissect the core principles of OOP, providing insights into its benefits, and exploring how it differs from other programming paradigms.
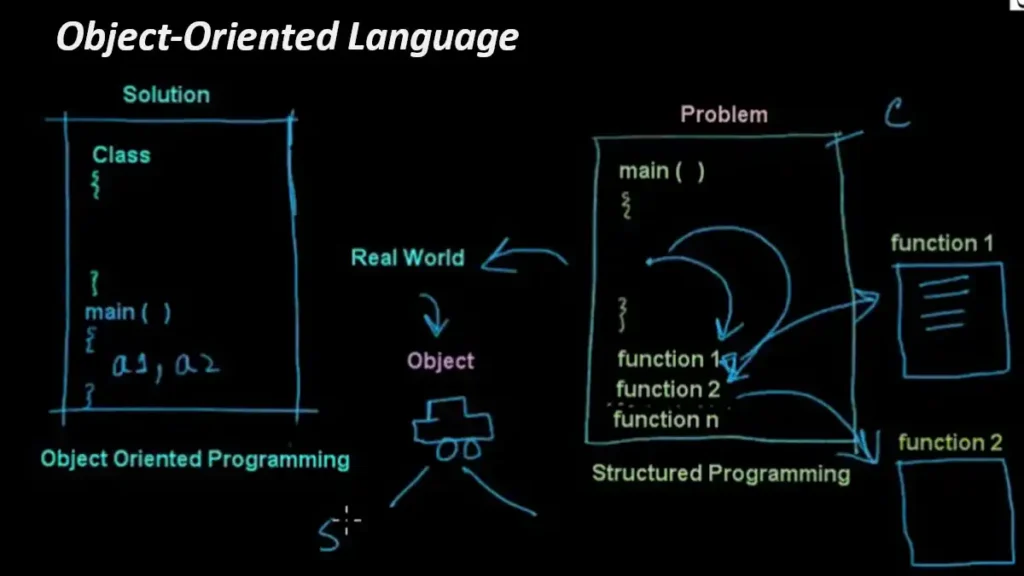
What is an Object-Oriented Language (OOL)?
At its heart, an object-oriented language facilitates the practice of object-oriented programming (OOP) – a paradigm where software is structured around ‘objects’ rather than ‘actions’. These objects can be thought of as digital representations of tangible entities or abstract concepts. Each object possesses attributes (often referred to as properties or fields) and behaviors (referred to as methods). For example, consider a “Car” object. It may have attributes like “color”, “model”, and “speed”, and behaviors like “accelerate” and “brake”.
The primary goal of an Object Oriented Language is to bind together the data (attributes) and the functions that operate on the data (methods) into a single unit known as an object. This bundling ensures that an object maintains its state and can act on that state via its methods. By emphasizing this encapsulation, inheritance, polymorphism, and abstraction, Object Oriented Languages promote a more modular and organized approach to software design, making systems more scalable, maintainable, and intuitive.
» You should also read: What is an API (Application Programming Interface)?
Historical Overview of OOL: Tracing back the roots of object-oriented design
Before the arrival of object-oriented languages, developers primarily leaned on procedural languages. These languages were about executing a series of procedures or routines, which made the flow of programs linear and action-focused. However, as software requirements grew more intricate and systems became increasingly multifaceted, the need for a more organized, maintainable approach became evident. Enter Object-Oriented Design.
1960s
The genesis of the object-oriented concept dates back to the 1960s. The first significant stride in this realm was the programming language “Simula”, developed in the mid-1960s by Ole-Johan Dahl and Kristen Nygaard of Norway’s Oslo University. Intended for simulation, Simula introduced the notion of classes, instances, and inheritance. While it wasn’t extensively used beyond academia, Simula planted the seeds for future OOLs.
1970s
Then in the 1970s, Alan Kay’s team at Xerox PARC introduced “Smalltalk”, which became the archetype for modern object-oriented languages. Smalltalk not only refined the ideas from Simula but also introduced the powerful idea of encapsulation and messaging between objects. It was in Smalltalk that the complete ecosystem of classes, objects, inheritance, and polymorphism coalesced into a cohesive model.
1980s / 1990s
The 1980s and 1990s witnessed an explosion of object-oriented languages, with C++ (an extension of the C language), Java, Python, and Ruby rising in prominence. Each brought its own flavor and contributed significantly to the advancement of OOP principles, making it the dominant programming paradigm in the contemporary software development landscape.
Core Pillars of OOP: An in-depth look at Encapsulation, Inheritance, Polymorphism, and Abstraction
Encapsulation:
Encapsulation is often analogized as the protective shield that prevents random access to certain details of an object. In technical terms, it’s about binding the data (variables) and the methods (functions) that operate on the data into a single entity known as an object. Moreover, it restricts direct access to some of the object’s components, which is a fundamental tenet of the ‘black-box’ concept in software engineering. This not only safeguards the integrity of the data but also makes the software more robust and modifiable.
Inheritance:
Inheritance allows developers to create a new class based on an existing class. The new class inherits attributes and behaviors (methods) from the parent class. This promotes code reusability and establishes a natural hierarchy between objects. For instance, in a system simulating animals, a ‘Bird’ class could inherit attributes and methods from a more general ‘Animal’ class, while also introducing new methods like ‘fly’.
Polymorphism:
Derived from Greek words meaning “many shapes”, polymorphism allows objects of different classes to be treated as objects of a common superclass. At its core, it’s about one interface, multiple methods. For instance, a ‘draw’ method might be implemented differently for ‘Triangle’, ‘Circle’, or ‘Rectangle’ classes, yet a user can call the ‘draw’ method on any shape without knowing which specific shape it is. This dynamic method invocation ensures flexibility and makes systems more extensible.
Abstraction:
Abstraction is the art of hiding complex implementation details and showing only the essential features of an object. It allows programmers to hide the complex reality while exposing only the necessary parts. Think of it like a car dashboard. A driver doesn’t need to understand the intricate details of how the engine works. They only need to know how to interact with the dashboard’s controls – the abstraction of the car’s functionalities.
Together, these four pillars form the bedrock of object-oriented programming, enabling developers to craft software that’s scalable, maintainable, and aligned with the way humans naturally perceive the world.
» Don’t miss our incredible article about Quantum Computing!
Comparison with Procedural Languages: Highlighting the distinctions between OOP and procedural programming
Object-oriented programming (OOP) and procedural programming are two of the most prominent paradigms in the software development world. While both have their merits, understanding their key differences is essential for a well-rounded grasp of computer science concepts.
Design Philosophy:
- OOP: Focuses on data and the methods that can be applied to that data. It promotes the concept of code as real-world entities or objects.
- Procedural: Centers around the idea of procedure calls where you typically have routines that operate on data, making the flow of the program linear and top-down.
Organization:
- OOP: Code is organized around objects and classes, creating a blueprint that can be instantiated multiple times.
- Procedural: Code is organized around functions, and data is typically passed between functions.
Data Access:
- OOP: Encapsulation restricts unauthorized operations on the data. Objects control the data and offer methods to access or modify them.
- Procedural: Data is more openly accessible. Any routine can access and modify data if it’s in scope.
Scalability and Maintenance:
- OOP: Easier to scale and maintain, especially for large applications. The modular nature of objects means a change in one object doesn’t necessarily affect others.
- Procedural: Can become complex and hard to maintain as programs grow. A small change may force changes in multiple areas.
Isn’t OOP Less Efficient?
It’s a common misconception that OOP is inherently less efficient than procedural programming. While OOP may introduce some overhead due to the abstraction layers, it doesn’t necessarily make it slower. In fact, in many real-world scenarios, the maintainability, clarity, and modular nature of OOP can lead to more optimized and efficient code in the long run. However, for certain low-level operations or performance-critical applications, procedural programming might offer a slight edge in raw performance due to its direct and linear approach.
Real-world applications of OOP
The beauty of Object-Oriented Programming is its ability to model and solve complex problems in ways that align closely with how we perceive the real world. Here are some concrete examples of OOP in action:
GUI Applications:
Most of the modern graphical user interface (GUI) applications, like those built using Java’s Swing or Microsoft’s .NET Framework, are structured using OOP principles. Each element of the GUI (buttons, windows, text boxes) is an object with properties and methods.
Gaming Development:
Game characters, terrains, weapons, and even behaviors can be modeled as objects. Unity and Unreal Engine, two of the leading game development platforms, leverage OOP for game design.
Real-time Systems:
Consider an air traffic control system. Each airplane can be modeled as an object with properties like speed, altitude, and position. Methods can determine the distance between planes, potential collisions, and safe flight paths.
E-commerce Platforms:
Sites like Amazon or eBay model each product as an object with properties such as price, category, and seller. The operations like adding to cart, applying discounts, or calculating shipping costs are methods tied to these objects.
Database Systems:
Object-relational mapping (ORM) frameworks, like Hibernate in Java or Django’s ORM in Python, allow developers to work with databases using OOP. Here, database tables are represented as classes and records as objects.
Web Development Frameworks:
Frameworks like Ruby on Rails or Angular are built on OOP principles. Components or models in these frameworks are designed as objects, enhancing modularity and reusability.
These examples underscore the versatility of OOP. Whether you’re building a cutting-edge game, designing an intuitive UI, or streamlining a complex system like air traffic control, OOP provides a structured, intuitive, and scalable approach to solving problems.
Main and Most Used Object-Oriented Languages
Over the decades, many programming languages have adopted or been designed around the principles of object-oriented programming (OOP). These languages have often become the foundation for many of the software systems that power the modern world. Here’s a list of some of the most prominent object-oriented languages:
- Java: Originally designed for interactive television, it has grown to become one of the most widely used languages for web applications, mobile apps (Android), and enterprise software.
- Python: Renowned for its simplicity and readability, Python’s versatility has led to its use in web development, artificial intelligence, data science, and more.
- C++: An extension of the C language, C++ added object-oriented features to the powerful capabilities of C, making it popular in systems/software development and game development.
- C# (C-Sharp): Developed by Microsoft, it’s a language for the .NET framework. Commonly used for developing Windows applications.
- Ruby: Known for its elegant syntax, it’s widely used in web development, especially with the Ruby on Rails framework.
- Objective-C: Primarily used by Apple for macOS and iOS development before Swift was introduced.
- Swift: Apple’s newer language for macOS, iOS, watchOS, and tvOS application development.
- Smalltalk: One of the earliest object-oriented languages and played a fundamental role in the development of the OOP paradigm.
- Scala: A hybrid functional/OOP language that runs on the Java virtual machine (JVM).
- PHP: While it started as a procedural language, newer versions have incorporated OOP features, and it’s a mainstay in web development.
These languages, though diverse in syntax and use-case, all encapsulate the core principles of object-oriented programming, enabling developers to design scalable, maintainable, and efficient software systems.
Code Examples: Object Creation, Properties, and Methods in Python
Python stands out for its simplicity and readability, making it an excellent choice for demonstrating OOP concepts.
Let’s dive into an example:
Defining a Class (Blueprint):
class Dog:
# Initializer / Constructor
def __init__(self, name, breed):
self.name = name # Property
self.breed = breed # Property
# Method
def bark(self):
return f"{self.name} barks loudly!"
# Method to set breed
def set_breed(self, breed):
self.breed = breed
Creating an Object (Instance):
# Creating a Dog object
my_dog = Dog(name="Buddy", breed="Golden Retriever")
Accessing Properties:
print(my_dog.name) # Output: Buddy
print(my_dog.breed) # Output: Golden Retriever
Calling Methods:
print(my_dog.bark()) # Output: Buddy barks loudly!
Modifying Properties with a Method:
my_dog.set_breed("Labrador")
print(my_dog.breed) # Output: Labrador
In this Python example, we defined a Dog class with properties like name and breed. We also defined methods like bark and set_breed. Once our class was defined, we created an instance of it (my_dog) and interacted with its properties and methods. This encapsulation of data and behavior into a single entity, an object, is the essence of object-oriented programming.
Essential Reading: Comprehensive Books on Object-Oriented Programming
Diving deeper into object-oriented programming can be both exciting and overwhelming, but the journey can be made smoother with the right resources. Here’s a list of seminal books that have shaped, educated, and guided programmers in the realm of OOP:
- “Design Patterns: Elements of Reusable Object-Oriented Software” by Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides. This book discusses 23 classic software design patterns and is foundational for anyone serious about OOP.
- “Object-Oriented Analysis and Design with Applications” by Grady Booch. A classic that delves into the methodologies and practices of OOP.
- “The Object-Oriented Thought Process” by Matt Weisfeld. Suitable for beginners, this book provides a clear introduction to thinking in an object-oriented way.
- “Object Thinking” by David West. West delves deep into the philosophy behind OOP, helping programmers understand not just the how but the why.
- “Object Design: Roles, Responsibilities, and Collaborations” by Rebecca Wirfs-Brock and Alan McKean. A fantastic guide on designing objects with responsibilities and collaborations in mind.
- “Applying UML and Patterns: An Introduction to Object-Oriented Analysis and Design and Iterative Development” by Craig Larman. A blend of theory and practical application with UML and design patterns.
- “Object-Oriented Software Construction” by Bertrand Meyer. A comprehensive treatise on all things OOP.
Remember, while these books are highly recommended, the best way to truly understand OOP is by designing, coding, and refactoring real-world applications. Combine theory with practice for the most effective learning.
Conclusion
Object-oriented programming (OOP) revolutionized the way developers approach software design, offering a paradigm that closely mimics the real world with its emphasis on objects and their interactions. It’s a model that promotes modularity, reusability, and maintainability. While it’s not without its critics or considerations, especially concerning efficiency in certain applications, its benefits often outweigh its drawbacks, especially in large-scale application development.
The contrast with procedural languages, its historical journey, the core principles, and its implementation in major programming languages demonstrates the vastness and depth of Object Oriented Languages. Like any tool or methodology, it’s about using it judiciously, understanding its strengths and limitations, and continuously learning. As the software world evolves, OOP remains a robust and reliable stalwart, proving its worth time and again.
For those eager to explore further, the world of object-oriented design and programming is vast, with countless resources, communities, and experts eager to share knowledge. Whether you’re a budding programmer or an experienced developer, the journey with OOP is one of continuous discovery and growth.