Load balancing is one of those topics that sits at the heart of high-availability systems, cloud architecture, and scalable applications. While several algorithms offer nuanced ways of distributing workloads across multiple resources, few are as straightforward and widely adopted as the Round Robin Algorithm. It’s like the bread and butter of load balancing—a staple in the repertoire of system administrators and network engineers.
In this article:
- What is the Round Robin Algorithm?
- Technical Breakdown
- Strengths and Weaknesses
- Weighted Round Robin
- Round Robin in DNS
- Round Robin vs. Other Algorithms
- Real-World Case Studies
- The Future of Round Robin
- Further Readings and Resources
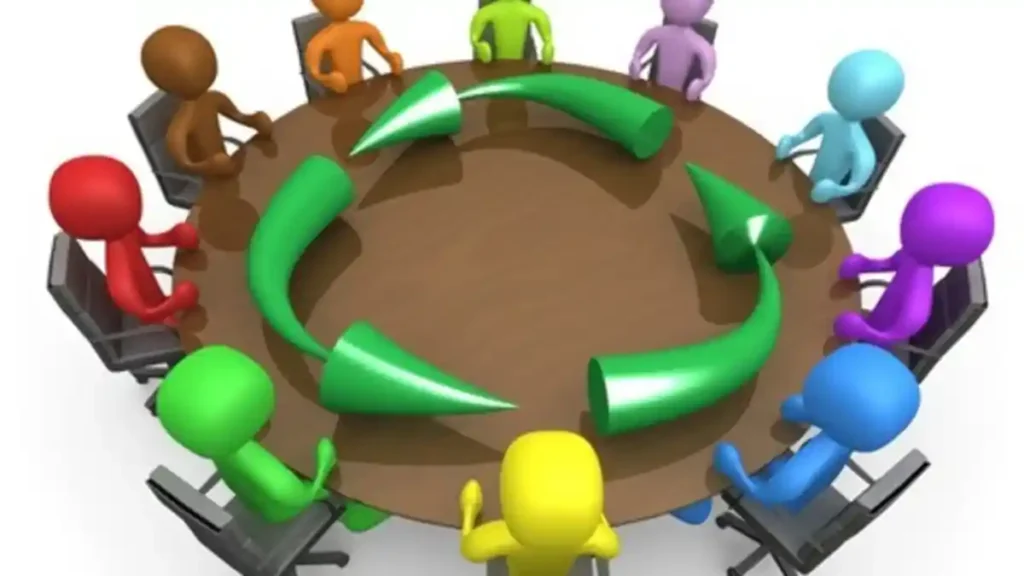
Round Robin is revered for its simplicity, but that doesn’t mean it lacks depth. From its basic working mechanism to its role in more complex setups, this algorithm has various facets worth exploring. Whether you’re an aspiring computer scientist, a seasoned network engineer, or someone just curious about how high-traffic websites seem to never go down, this article aims to offer a comprehensive understanding of the Round Robin Algorithm.
What is the Round Robin Algorithm?
The Round Robin Algorithm is a load-balancing technique that distributes incoming tasks or network requests across a pool of available servers in a cyclical manner. Imagine a queue of incoming requests and a circle of servers; the first request goes to the first server, the second request to the second server, and so on. When the last server in the circle is reached, the algorithm loops back to the first server, thus completing the “round.”
The Round Robin Algorithm is not resource-aware; it doesn’t consider the current load or capacity of a server while routing requests. This makes the algorithm simple to implement but also brings forth certain limitations when dealing with heterogeneous resources with varying capacities or workloads.
The appeal of Round Robin lies in its fairness and absence of favoritism—each server gets an equal opportunity to serve requests. However, this democratic approach may not always be the most efficient, especially when the capabilities of servers differ significantly.
Technical Breakdown
At its core, the Round Robin Algorithm is a cyclic enumerator that traverses a list of servers, ensuring each one gets its “turn” to serve incoming requests. Its beauty lies in its simplicity: it doesn’t require knowledge about the system’s internal workings or the server’s current load. This makes it both easy to implement and yet not always the most efficient choice, especially in heterogeneous environments where server capabilities differ.
Pseudocode for Basic Round Robin
To better understand Round Robin, let’s consider a straightforward pseudocode example:
In this pseudocode:
Initialize an empty queue of requests: request_queue
Initialize an array of servers: server_list
Initialize a pointer: server_pointer = 0
While request_queue is not empty:
Dequeue a request from request_queue: current_request
Assign current_request to server_list[server_pointer]
server_pointer = (server_pointer + 1) mod server_list.length
request_queue
holds the incoming requests.server_list
holds the available servers.server_pointer
points to the next server inserver_list
that will get the incoming request.
Each time a new request comes in (current_request
), it gets assigned to the server pointed to by server_pointer
. The pointer then increments and wraps around if it reaches the end of the server list.
Key Characteristics
- Cyclic Nature: Round Robin cycles through the list of servers, going back to the start when it reaches the end.
- Statelessness: Each decision is stateless, meaning it does not depend on past allocations.
- Fairness: Every server gets an equal chance, ensuring a fair distribution but not necessarily the most efficient one.
- Simplicity: The algorithm doesn’t require complex computations, making it lightweight and fast.
Time Complexity
The time complexity of a basic Round Robin Algorithm is O(1) for each operation. This means that the algorithm can make a decision in constant time, irrespective of the number of servers or incoming requests. The computational cost is negligible, making Round Robin an extremely fast algorithm, albeit not always the most resource-efficient.
Limitations and Nuances
While the algorithm is straightforward, its indiscriminate nature means it doesn’t take into account the current load or capacity of a server. In scenarios where servers have differing capabilities or are already under heavy load, Round Robin can be inefficient.
To illustrate, if Server A can handle 100 requests per second, and Server B can only handle 50, using a simple Round Robin approach would result in an imbalanced load. Weighted Round Robin, a variant of the algorithm, addresses this issue by assigning more turns to more capable servers.
In summary, the Round Robin Algorithm is an elegantly simple yet powerful technique for distributing workloads across multiple servers. It’s easy to implement, and fast to execute, but also naive in its distribution, making it a good fit for specific scenarios but not a one-size-fits-all solution.
Strengths and Weaknesses
Strengths
- Simplicity: One of the key advantages of the Round Robin Algorithm is its simplicity. It’s straightforward to implement, which makes it accessible to engineers of all levels.
- Quick Decision Making: Thanks to its O(1) time complexity for decision-making, Round Robin is extremely quick. This enables it to serve large numbers of requests with minimal delay.
- Fairness: The algorithm is designed to distribute incoming tasks evenly across all servers, ensuring that no single server is overwhelmed while others are idle.
- Statelessness: Round Robin is stateless, meaning it doesn’t keep track of the past. This attribute simplifies implementation and minimizes the overhead associated with maintaining state information.
- Low Overhead: Due to its straightforward nature and constant-time operation, Round Robin doesn’t require heavy computational power, making it resource-efficient in that regard.
Weaknesses
- Lack of Resource Awareness: Round Robin doesn’t consider the current load or capabilities of a server when assigning tasks, which can lead to inefficiency.
- Not Ideal for Varied Workloads: In a system where tasks may require vastly different computational resources, Round Robin’s equal distribution mechanism may lead to bottlenecks.
- Possible Imbalance: In the real world, not all servers are created equal. Some may be more powerful than others. Round Robin doesn’t account for this, often resulting in resource imbalance.
- Limited Fault Tolerance: The algorithm doesn’t inherently offer a way to skip or replace a failed server, making it necessary to incorporate additional mechanisms for fault tolerance.
- No Priority Assignment: Round Robin treats all requests as equals, which may not be ideal for systems that need to prioritize certain types of tasks.
Weighted Round Robin
In environments where servers have differing capabilities, a basic Round Robin approach can lead to inefficiencies. Enter Weighted Round Robin—a modified version of the algorithm that aims to distribute tasks based on server capacity or some other pre-defined metric.
How Does Weighted Round Robin Work?
In Weighted Round Robin, each server is assigned a weight, an integer that signifies the server’s capacity or priority. The algorithm ensures that servers with higher weights receive a proportionally higher number of tasks.
Pseudocode for Weighted Round Robin
Initialize an empty queue of requests: request_queue
Initialize an array of servers with weights: weighted_server_list
Initialize a pointer: server_pointer = 0
Initialize total_weight: sum(weights in weighted_server_list)
While request_queue is not empty:
Dequeue a request from request_queue: current_request
Assign current_request to server in weighted_server_list based on cumulative weight
Update server_pointer based on server's weight and total_weight
In this pseudocode, weighted_server_list
is an array where each server entry has an associated weight, and total_weight
is the sum of all server weights. The algorithm selects a server based on the cumulative weight, which involves some calculation as opposed to the straightforward nature of basic Round Robin.
Use-Cases
Weighted Round Robin is particularly useful in:
- Cloud Environments: Where virtual machines may have different capacities.
- Multi-Tier Architectures: Where different layers of the application may have different processing capabilities.
- Content Delivery Networks: Where some nodes may be geographically closer to the end-user, and you might want to weight them higher to reduce latency.
Limitations
While Weighted Round Robin addresses the naivety of basic Round Robin, it adds complexity, requiring a method to assign and potentially update weights. Additionally, it still shares some limitations with its simpler counterpart, such as limited fault tolerance.
By understanding both the advantages and disadvantages of Round Robin and its weighted variant, system architects can make more informed decisions on which load balancing algorithm to employ in different scenarios. As always, the key is to align the algorithm’s attributes with the system’s specific needs and constraints.
Round Robin in DNS
The Domain Name System (DNS) often employs Round Robin as a rudimentary load balancing mechanism. Unlike more specialized load balancers that operate at the network or application layers, DNS servers usually have a simpler role: mapping domain names to IP addresses. When a domain resolves to multiple IP addresses, DNS can distribute incoming requests by rotating the order of the IP address records it returns. This is DNS-based Round Robin in action.
How It Works
- Multiple A Records: A domain has multiple A or AAAA records, each pointing to a different server IP address.
- Cyclic Rotation: Each time a DNS request is made, the DNS server rotates the list of IPs before sending it to the client.
- Client-side Handling: The client generally connects to the first IP in the list, thus effectively distributing the load among servers.
Limitations in DNS Context
- Caching: DNS responses are often cached at multiple levels, from the operating system to intermediate DNS resolvers. This caching behavior can undermine the distribution efficacy.
- No Health Checks: DNS doesn’t perform health checks on the servers, which means it could point to a server that is down.
- Geo-Location Inefficiencies: DNS-based Round Robin doesn’t consider the geographic location of the client or server, potentially leading to latency issues.
Round Robin vs. Other Algorithms
Least Connections
The Least Connections algorithm dynamically allocates each new request to the server with the fewest active connections.
- Resource Awareness: Unlike Round Robin, Least Connections considers server load, aiming for a more efficient resource utilization.
- Complexity: This algorithm requires tracking the number of active connections for each server, adding a layer of complexity.
- Time-Sensitivity: Least Connections is effective when the service time varies significantly between requests.
IP Hash
The IP Hash algorithm maps each client IP address to a server, ensuring that all requests from a given client are sent to the same server.
- Session Persistence: Ideal for applications requiring session persistence, as it guarantees that a client is consistently mapped to the same server.
- Limited Distribution: The algorithm can lead to imbalances if a few client IPs generate a high number of requests.
- Predictability: This approach is deterministic but lacks the dynamism to adapt to changing server loads.
Comparative Summary
- Simplicity: Round Robin wins in simplicity but lacks in resource awareness.
- Resource Allocation: Least Connections excels in efficient resource allocation but involves additional overhead.
- Session Stickiness: IP Hash is most useful when session stickiness is required but may lead to imbalances.
- Flexibility: Round Robin is highly flexible, whereas Least Connections and IP Hash require more context and state management.
Understanding the nuances of these algorithms helps system architects and developers make educated decisions when facing diverse operational requirements. While Round Robin remains a strong contender due to its simplicity and fairness, specialized environments might benefit from the resource awareness of Least Connections or the session persistence of IP Hash. As with most technical choices, the “best” option is highly context-dependent.
Real-World Case Studies
Web Server Load Balancing
One of the most straightforward applications of Round Robin is in web server load balancing. Companies like Netflix and Amazon have utilized Round Robin in some form, especially in the early phases of their growth, to distribute incoming HTTP requests across multiple servers.
DNS Load Balancing in Content Delivery Networks
CDNs like Akamai and Cloudflare often use DNS-based Round Robin to distribute user requests to geographically distributed cache servers. This helps in lowering latency and improving user experience, especially for static content.
Kubernetes and Container Orchestration
Kubernetes, the leading container-orchestration system, employs a form of Round Robin for its basic service discovery. Though Kubernetes supports more advanced load balancing techniques, Round Robin remains an option due to its simplicity.
Limitations and Adaptations
Each of these real-world applications has had to adapt or move beyond Round Robin to address its limitations. For example, Kubernetes now often pairs it with other algorithms or even AI-based load balancers to achieve better resource utilization.
The Future of Round Robin
As we move into an era of cloud-native applications, microservices, and serverless architectures, the relevance of Round Robin can be questioned. However, its core principles of fairness and simplicity make it a good starting point for any load balancing strategy. More advanced algorithms and load balancers often incorporate Round Robin as one of the strategies in a multi-algorithm approach.
Evolving Algorithms
Round Robin will likely not be the final say in load balancing. Algorithms based on machine learning and predictive analytics are entering the scene, and they promise more efficient resource utilization by dynamically adapting to changes in system state and workload.
Round Robin in Hybrid Systems
The future may see Round Robin integrated into more complex, AI-driven systems as a fallback mechanism, or as part of a hybrid strategy that uses Round Robin for initial distribution and other algorithms for fine-tuning.
Further Readings and Resources
Books
- “Load Balancing Servers, Firewalls, and Caches” by Chandra Kopparapu
- “Scalable Cloud Ops with Fugue” by Josha Stella, et al.
RFCs
- RFC 1794 – DNS Support for Load Balancing
- RFC 2782 – DNS RR for specifying the location of services (DNS SRV)