Windows PowerShell is an advanced, task-based command-line shell and scripting language designed for system administration. Built on the .NET framework, it enables administrators to more efficiently control and automate the administration of Windows operating systems and applications that run on Windows. This article aims to demystify Windows PowerShell for beginners, explaining its fundamentals, usage, and significance in modern computing.
PowerShell extends beyond traditional command-line shells. It offers a robust scripting environment, access to COM and WMI, and an integrated scripting editor—making it a powerful tool in the hands of system administrators and power users. By the end of this guide, you will have a foundational understanding of PowerShell, setting you up to explore its more advanced capabilities.
Table of Contents
- What is Windows PowerShell?
- Key Features and Capabilities
- PowerShell Scripting Basics
- How PowerShell Differs from Command Prompt
- Practical Applications and Examples
- Getting Started with PowerShell Commands
- References
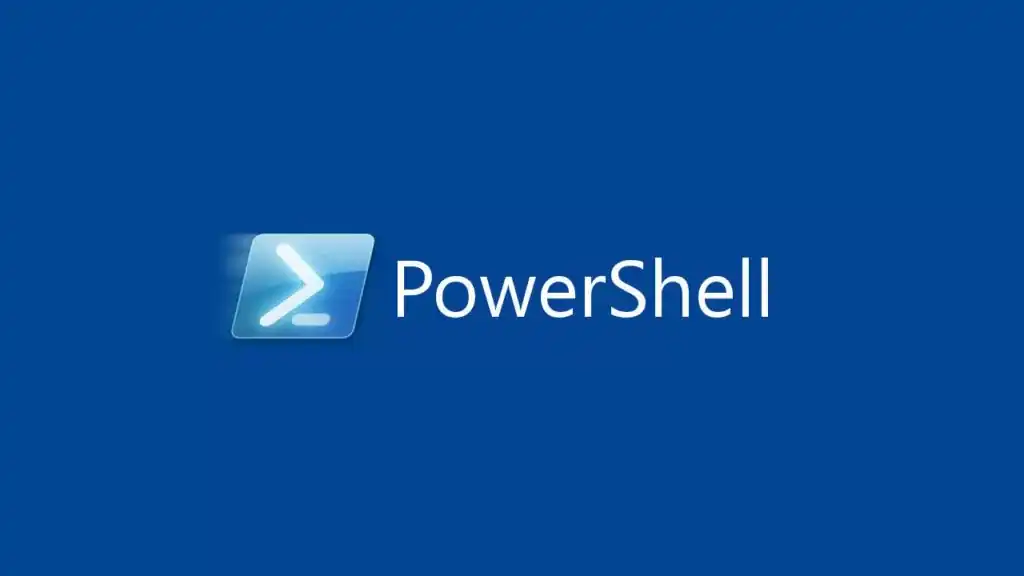
1. What is PowerShell?
Windows PowerShell is a command-line shell and scripting language developed by Microsoft. It was introduced in 2006 as a more powerful successor to the traditional Command Prompt in Windows. Designed primarily for system administration, PowerShell enables IT professionals to control and automate the administration of the Windows operating system and other applications.
At its core, PowerShell operates as both a command-line interface and a scripting language. It is built on the .NET Framework, allowing it to integrate with various Microsoft products seamlessly. This integration provides a comprehensive toolset for administrators to perform complex tasks through simple commands and scripts. PowerShell scripts are stored in .ps1
files, and they can automate tasks, configure systems, and manage resources.
One of the key strengths of PowerShell is its object-oriented nature, which means that it processes data as objects. This is a significant shift from traditional text-based shells, which deal primarily with text. This object-oriented approach provides more flexibility and power in manipulating data and performing operations. PowerShell also includes a rich set of cmdlets (command-lets), which are built-in commands, to perform common system administration tasks, making it an essential tool in the modern IT environment.
2. Key Features and Capabilities
Windows PowerShell stands out as a powerful tool in system administration due to its unique set of features and capabilities. Understanding these key aspects will help beginners appreciate the power and flexibility of PowerShell.
- Cmdlets and Modules: At the heart of PowerShell are cmdlets, specialized .NET classes that implement specific functions. These cmdlets can be used independently or combined to perform complex tasks. PowerShell modules, on the other hand, are packages of cmdlets, providers, functions, and workflows that add functionality to the PowerShell environment.
- Object-Oriented Nature: Unlike traditional command-line interfaces that output text, PowerShell operates on objects. An object in PowerShell is a data structure that represents an item in the system, like a file or a process. This object-oriented approach allows for more sophisticated data manipulation and control.
- Scripting Language: PowerShell is also a scripting language, allowing users to write scripts to automate complex tasks. These scripts can range from simple command sequences to complex programs with loops, conditions, and error handling.
- Pipeline Support: PowerShell’s pipeline support is a powerful feature that allows users to pass the output of one cmdlet as input into another cmdlet. This chaining of commands facilitates complex data operations with minimal scripting.
- Access to COM and WMI: PowerShell provides access to Component Object Model (COM) and Windows Management Instrumentation (WMI), enabling the management and automation of remote systems and environments.
- Integrated Scripting Environment (ISE): PowerShell ISE is a graphical host application that provides a multi-tabbed environment for running and debugging scripts, complete with syntax highlighting, tab completion, and context-sensitive help.
- Security Features: PowerShell includes robust security features like execution policies to control the running of scripts and the ability to sign scripts with a digital certificate.
- Remote Management: Through PowerShell remoting, administrators can run commands on remote systems, making it an invaluable tool for managing a network of computers.
- Community Support: A vibrant community and a vast collection of third-party scripts and modules expand PowerShell’s functionality and support continual learning.
3. PowerShell Scripting Basics
PowerShell’s scripting capabilities are a cornerstone of its functionality. Here are some basic concepts and examples to help beginners start scripting in PowerShell.
Basic Script Structure
A PowerShell script is a plain text file containing PowerShell commands, saved with a .ps1
file extension. Scripts can include commands, functions, loops, variables, and more.
Example 1: Hello World Script
# This is a simple script to display a message
Write-Host "Hello, World!"
Variables and Data Types
PowerShell scripts can use variables to store data. PowerShell is object-oriented, so variables can store not just strings or numbers, but also complex objects.
Example 2: Using Variables
# Define a variable
$greeting = "Welcome to PowerShell"
# Display the value of the variable
Write-Host $greeting
Conditional Statements
Conditional statements, like if-else
, allow scripts to execute code based on conditions.
Example 3: If-Else Statement
# Check if a number is greater than 10
$number = 15
if ($number -gt 10) {
Write-Host "The number is greater than 10."
} else {
Write-Host "The number is 10 or less."
}
Loops
Loops are used to repeat actions. For instance, the foreach
loop is useful for iterating over items in a collection.
Example 4: Foreach Loop
# Loop through a list of numbers
$numbers = 1, 2, 3, 4, 5
foreach ($number in $numbers) {
Write-Host "Number: $number"
}
Functions
Functions in PowerShell are blocks of code that perform a specific task and can be reused in scripts.
Example 5: Function
# Define a function
function Say-Hello {
param ($name)
Write-Host "Hello, $name!"
}
# Call the function
Say-Hello -name "Alice"
Error Handling
PowerShell supports error handling using try-catch-finally
blocks, allowing scripts to gracefully handle unexpected issues.
Example 6: Error Handling
# Try-Catch block for error handling
try {
# Code that might cause an error
$result = 1 / 0
} catch {
Write-Host "An error occurred: $_"
}
These examples provide a glimpse into PowerShell scripting. As you practice and explore more, you’ll discover the full potential of PowerShell in automating and managing tasks.
4. How PowerShell Differs from Command Prompt
Understanding the differences between PowerShell and the traditional Windows Command Prompt (CMD) is crucial for beginners. While both are command-line interfaces used for various tasks in Windows, they have distinct differences in capabilities, design, and usage.
- Command Syntax and Capabilities: CMD uses a simple command syntax and is primarily used for executing batch scripts and system utilities. PowerShell, however, offers a more complex syntax and is designed for system administration, offering a wide range of cmdlets for managing the Windows environment.
- Object-Oriented Output: CMD processes and outputs data as text. PowerShell, in contrast, works with objects. When a command is executed in PowerShell, it returns objects with properties and methods that can be further manipulated, providing a more granular control.
- Scripting Language Features: PowerShell is not only a command-line shell but also a full-fledged scripting language. It supports complex scripts with advanced features like functions, loops, and error handling, which are beyond the capabilities of CMD’s batch scripting.
- Access to .NET Framework: PowerShell is built on the .NET Framework, allowing it to access and utilize a vast range of .NET classes and libraries. This enables more sophisticated and powerful operations compared to CMD.
- Remote Management: PowerShell supports remote management, allowing administrators to execute commands and scripts on remote computers. This is a significant advantage over CMD, which has limited capabilities in remote management.
- Pipeline Feature: PowerShell’s pipeline feature allows chaining of commands, where the output of one command can be passed as input to another. This feature is more advanced compared to CMD’s simpler piping capabilities.
- Integrated Scripting Environment (ISE): PowerShell includes an Integrated Scripting Environment (ISE), a graphical user interface that provides a rich scripting experience with features like syntax highlighting and debugging. CMD does not offer a similar integrated environment.
5. Practical Applications and Examples
Windows PowerShell is a versatile tool with numerous practical applications. Here are some common use cases and examples that showcase its utility:
- System Administration Tasks: Automating routine tasks like user account management, disk space monitoring, and software installation.Example: Automating user creation
New-ADUser -Name "John Doe" -SamAccountName "jdoe" -UserPrincipalName "jdoe@domain.com"
- File and Directory Operations: Managing files and directories more efficiently, including batch renaming, searching for files, and modifying file attributes.
Example: Bulk renaming files
Get-ChildItem "C:\Documents\*.txt" | Rename-Item -NewName { $_.Name -replace '.txt','.log' }
- Network Management: Managing network configurations, analyzing network statistics, and automating network diagnostics.
Example: Checking network connectivity
Test-Connection google.com -Count 5
- Registry and System Configuration: Accessing and modifying the Windows Registry and system settings for configuration management.
Example: Changing registry values
Set-ItemProperty -Path "HKLM:\Software\MyCompany\MyApp" -Name "SettingName" -Value "NewValue"
- Process and Service Management: Monitoring and controlling system processes and services.
Example: Restarting a Windows service
Restart-Service -Name "Spooler"
- Automating Complex Workflows: Creating scripts that combine multiple tasks into a single, automated workflow for efficiency.
Example: Backup and compress a directory
Compress-Archive -Path C:\Data -DestinationPath C:\Backup\DataBackup.zip
6. Getting Started with PowerShell Commands
For beginners, the best way to start with PowerShell is to familiarize yourself with some basic commands and their syntax. Here’s a guide to get you started:
- Viewing Commands (Cmdlets): Use
Get-Command
to list all available cmdlets in PowerShell.
Get-Command
- Getting Help: To understand how to use a cmdlet, use
Get-Help
.
Get-Help Get-Process
- Managing Files and Directories: Use cmdlets like
Get-ChildItem
,New-Item
, andRemove-Item
to work with files and directories.
Get-ChildItem -Path C:\Documents
- Working with Processes: Use
Get-Process
to view running processes orStop-Process
to terminate a process.
Get-Process
Stop-Process -Name Notepad
- Managing Services: Use
Get-Service
to list services orStart-Service
andStop-Service
to control service states.
Get-Service | Where-Object {$_.Status -eq "Running"}
- System Information: Use cmdlets like
Get-SystemInfo
to retrieve detailed system information.
Get-SystemInfo
- Creating and Running Scripts: Scripts are written in plain text and saved with a
.ps1
extension. To run a script, type its path in the PowerShell console.
C:\Scripts\MyScript.ps1
By practicing these commands and exploring further, you will gradually become proficient in using PowerShell for a variety of tasks and automation. Remember, the key to mastering PowerShell is regular practice and exploration of its extensive documentation and community resources.
7. References
- Books:
- “Windows PowerShell Cookbook” by Lee Holmes
- “Learn Windows PowerShell in a Month of Lunches” by Donald W. Jones and Jeffrey Hicks
- “PowerShell in Depth: An Administrator’s Guide” by Don Jones, Jeffrey Hicks, and Richard Siddaway
- Websites: