The Telephony Application Programming Interface, commonly known as TAPI, is a critical standard for interfacing telephony services in Microsoft Windows. Developed in collaboration between Microsoft and Intel, TAPI enables applications to control and manage telephony functions. This article delves into what TAPI is, how it works, and why it’s important for modern and legacy applications alike.
Table of Contents:
- What is TAPI (Telephony Application Programming Interface)?
- How TAPI Interface Works
- TAPI 2.x vs TAPI 3.x: A Comparative Analysis
- TAPI Locations: A Use-Case
- TAPI Interface in Modern Telephony
- References
1. What is TAPI (Telephony Application Programming Interface)?
TAPI is a set of standard application programming interfaces (APIs) for accessing telephony services developed by Microsoft and Intel and implemented in Microsoft Windows.
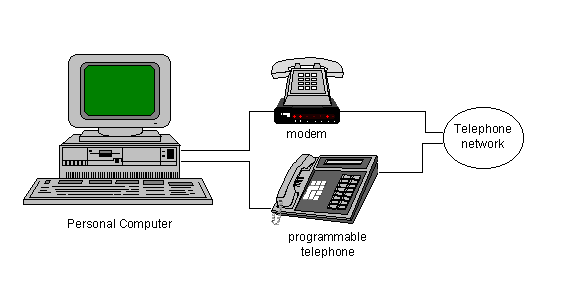
TAPI, or Telephony Application Programming Interface, is an application programming interface (API) standard tailored for Windows operating systems. Developed through a joint effort between Microsoft and Intel, TAPI provides a uniform way of controlling and managing telephony devices, like modems, VoIP phones, ISDN terminals, and Private Branch Exchanges (PBXs), through software applications.
The Genesis of TAPI
TAPI was conceptualized in the 1990s to create a bridge between computer applications and telephony hardware. Before TAPI, there was a lack of standardized methods to interact with different telephony hardware, making it cumbersome for software developers to create applications that could work across multiple devices.
Unified Communications
While TAPI began with a focus on traditional telephony, it has evolved to play a role in unified communications. The API standard can handle not just voice, but also data and video communication, which is vital in the era of Zoom, Teams, and other unified communications platforms.
Example Code for Initiating a TAPI Call
Here’s a simple example in C# to demonstrate how a TAPI-compliant application might initiate a call.
using TAPI3Lib;
...
private void MakeCall(string phoneNumber)
{
TAPIClass tapi = new TAPIClass();
tapi.Initialize();
ITAddress address = tapi.Addresses[0];
ITBasicCallControl call = address.CreateCall(phoneNumber, TapiConstants.TAPIMEDIATYPE_AUDIO, TapiConstants.TAPIADDRESSMODE_ADDRESS);
call.Connect(false);
}
2. How TAPI Interface Works: Under the Hood
TAPI Interface functions as a middleman between software applications and telephony hardware. It receives high-level requests from applications and translates them into low-level instructions that the hardware understands.
The Request and Forward Mechanism
When an application needs to use telephony services, it makes a request to the TAPI service. This request could be anything from dialing a number to setting up a complex multi-point conference call. TAPI processes this request and forwards it to the telephony hardware which then carries out the action.
Code Sample: Handling Incoming Calls
In a TAPI application, handling an incoming call might look something like this in C#:
public void HandleIncomingCall(ITCallNotificationEvent e)
{
ITBasicCallControl incomingCall = (ITBasicCallControl)e.Call;
incomingCall.Answer();
}
TAPI and Device Drivers
One of the reasons TAPI is so effective is that it abstracts the complexities of interacting directly with telephony hardware. Device drivers specific to the telephony hardware interact with TAPI, allowing for a more unified coding experience for developers.
Example Code for Hanging Up a Call
Here’s an example to hang up a call using TAPI in C#:
private void HangUp(ITBasicCallControl call)
{
call.Disconnect(TapiConstants.TAPIDISCONNECTMODE_NORMAL);
}
3. TAPI 2.x vs TAPI 3.x: A Comparative Analysis
Understanding the versions of TAPI is crucial for choosing the right telephony services for your specific application needs. Although both TAPI 2.x and TAPI 3.x serve the same core purpose, they differ significantly in their features, capabilities, and underlying architecture.
TAPI 2.x: The Foundation
TAPI 2.x is the earlier version that was widely adopted in various applications. It is a COM (Component Object Model)-based API and offers a lower-level interface compared to TAPI 3.x. It provides robust support for traditional telephony devices and works exceptionally well for simpler applications that don’t require advanced features.
Features of TAPI 2.x
- COM-based API.
- Lower-level interface for greater control.
- Robust support for older telephony services and hardware.
TAPI 3.x: The Evolution
TAPI 3.x was introduced to adapt to the modern needs of telephony and unified communications. Built on the COM+ architecture, it offers more advanced features, such as support for IP telephony and multimedia services. It provides a higher-level, simplified interface, making it easier to work with.
Features of TAPI 3.x
- COM+ based API.
- Higher-level, more abstracted interface.
- Support for IP telephony and multimedia services.
Code Comparison: Dialing a Number
TAPI 2.x Example in C++
ITBasicCallControl * pCall;
pAddress->CreateCall(bstrDestAddress, LINEADDRESSTYPE_PHONENUMBER, TAPIMEDIATYPE_AUDIO, &pCall);
pCall->Connect(VARIANT_FALSE);
TAPI 3.x Example in C#
ITAddress address = tapi.Addresses[0];
ITBasicCallControl call = address.CreateCall(phoneNumber, TapiConstants.TAPIMEDIATYPE_AUDIO, TapiConstants.TAPIADDRESSMODE_ADDRESS);
call.Connect(false);
Key Differences
- TAPI 3.x provides easier integration with modern technologies like IP telephony.
- TAPI 2.x gives finer control over hardware, which is useful for applications with specific needs.
- TAPI 3.x has built-in support for multimedia services, whereas TAPI 2.x is more focused on traditional voice communication.
By understanding the different versions of TAPI, developers can make an informed choice depending on their application requirements and the telephony hardware they intend to interface with.
4. TAPI Locations: A Use-Case
The concept of TAPI locations is particularly beneficial for frequent travelers or those using laptops across different geographical locations. A TAPI location is essentially a set of configurations that include country-specific dialing rules, area codes, and sometimes even specific calling card information. These pre-configured settings allow TAPI-aware applications to correctly dial numbers based on the user’s current location.
How to Create a TAPI Location
Creating a TAPI location involves specifying the following details:
- Country: The country you are currently in or will be traveling to.
- Area Code: The local area code for your location.
- Dial-Out Information: Any specific numbers that must be dialed to access an outside line.
- Calling Card Information: If you use a calling card for long-distance calls, this information can also be stored.
Once these settings are configured, TAPI-aware applications, such as dial-up networking programs or custom telephony applications, can utilize this information to make accurate calls.
Practical Applications
- Remote Work: TAPI locations are beneficial for remote workers who frequently switch locations but still require reliable telecommunication.
- Traveling Sales Teams: Sales personnel traveling to different cities or countries can switch TAPI locations to ensure correct dialing formats.
5. TAPI Interface in Modern Telephony
Though TAPI was introduced in a world dominated by landlines and simple cellular phones, its architecture has been continually updated to remain relevant in modern telephony. Today, TAPI is used to interface with a variety of newer technologies, including VoIP services and even unified communication systems.
Adaptability to IP Telephony
The most notable advancement is TAPI’s adaptability to IP telephony. With features in TAPI 3.x supporting IP-based communication, developers can seamlessly integrate modern telecommunication services into their applications.
Multimedia Services
TAPI is no longer restricted to audio-based telephony. The newer versions support multimedia services, enabling features like video conferencing and data transfer over the same communication lines.
Cloud Telephony
With the advent of cloud-based communication services, TAPI interfaces can be used to connect to these cloud services, providing businesses with scalable and flexible telecommunication solutions.
The Future of TAPI in Modern Telephony
While newer APIs and communication protocols are emerging, the widespread adoption and robustness of TAPI ensure its place in the modern telecommunication landscape, at least for the foreseeable future.
In sum, by understanding TAPI’s adaptability and how it interfaces with modern telecommunication technologies, organizations, and developers can better navigate their choices in today’s complex and ever-changing communication ecosystem.