Assembly language is a low-level programming language that provides a unique perspective on computer operations, sitting just above machine code in complexity. It is tailored specifically to the architecture of the computer being used, allowing programmers to manipulate hardware directly through a series of mnemonic codes that correspond to machine-level instructions. In this article, we’ll explore the rich history of assembly language, its practical applications, and the fundamental skills needed to begin programming in this precise language. Additionally, we’ll delve into why mastering assembly can be a significant advantage in various technical domains.
Index
- What is Assembly?
- The History of Assembly Language
- Key Applications of Assembly Language
- Benefits of Knowing Assembly Language
- Common Challenges in Assembly Programming
- Future of Assembly Language
- References
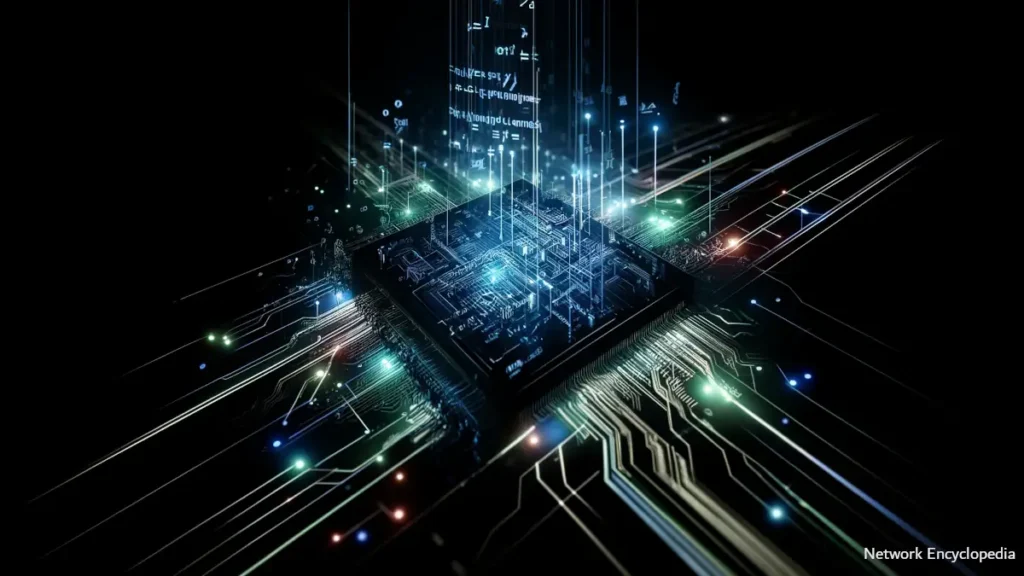
1. What is Assembly?
Assembly language, often simply called “assembly,” is a type of low-level programming language that is closely tied to the architecture of a computer’s hardware. Unlike high-level programming languages like Python or Java, which abstract away many of the complexities of the underlying hardware, assembly provides a direct line of communication with the machine’s components.
Why does this matter? In essence, coding in assembly allows developers to manipulate the system at a very granular level. This direct control can lead to optimized performance and more efficient use of resources. However, it also requires a deeper understanding of how computers actually work.
Assembly languages are specific to a particular computer architecture. This means that an assembly program written for an Intel x86 processor won’t work on an ARM processor, as each set of instructions is unique to the type of processor it was designed for. Because of its specific nature, assembly is often used in critical systems where speed and efficiency are paramount.
Assembly is not just about writing code; it’s about crafting it. Each line of an assembly program corresponds to a single operation in the computer’s CPU. As you write assembly code, you are effectively dictating the precise actions the CPU performs, step by step.
As we progress into more complex explanations, remember: assembly is the bedrock upon which software once relied heavily, and it still plays a critical role in many modern systems. By understanding assembly, you gain insight into the very heart of computing. Let’s delve deeper into the history and significant applications of assembly to appreciate its enduring relevance in the next chapters.
2. The History of Assembly Language
The invention of assembly language was a pivotal development in the evolution of computer programming. Before assembly language, programming was done directly in machine code, a series of binary digits that control a computer’s operations. This method was not only cumbersome but also highly prone to error.
In 1949, Kathleen Booth, a pioneering computer scientist, is credited with creating the concept of assembly language. Her work facilitated a more approachable form of coding, where symbolic instructions replaced binary code, making programming significantly more intuitive and less error-prone.
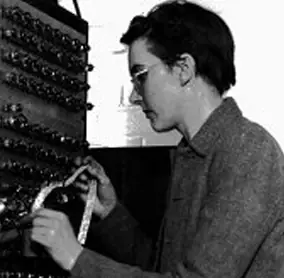
The first actual implementation of an assembler, a tool that translates assembly language into machine code, was developed by Nathaniel Rochester for the IBM 701 computer. This development marked a major step forward, enabling programmers to write more complex programs without delving into the intricacies of machine code.
Here’s a conceptual example of what an assembly language program might look like to perform a simple addition and store the result, annotated for clarity:
// Example Assembly Program for an IBM 701-like Architecture
// Purpose: Add two numbers and store the result
START: LOAD NUM1 // Load first number into accumulator
ADD NUM2 // Add second number to accumulator
STORE RESULT // Store result from accumulator to memory location RESULT
HALT // Stop the program
NUM1: DATA 5 // Define first number
NUM2: DATA 10 // Define second number
RESULT: DATA 0 // Memory space for result
// The program adds 5 and 10 and stores 15 in RESULT.
Throughout the 1950s and 1960s, assembly language became widely used, particularly in systems where performance and resource optimization were critical. Its ability to provide direct hardware control while being slightly easier to understand and debug than machine code made it ideal for the emerging needs of computing infrastructure, from military applications to the early space missions.
Today, while high-level languages dominate application development, the historical significance of assembly language lies in its foundational role in the software development hierarchy. It enabled the first complex software systems and operating systems to be written, setting the stage for modern computing.
3. Key Applications of Assembly Language
Despite the prevalence of high-level programming languages, assembly language still plays a critical role in various technological sectors. Its ability to provide precise control over system operations makes it indispensable in several key applications.
- Embedded Systems: In devices where resources are limited, such as microcontrollers used in home appliances, automotive electronics, and medical devices, assembly language allows developers to maximize efficiency and performance. It enables the software to run faster and use less memory, qualities essential in systems where adding more resources has physical and financial limitations.
- System Bootloaders: Assembly language is often used to write bootloaders, which initialize hardware and load operating systems when a computer starts. Its direct hardware interaction capabilities make it ideal for these foundational operations that precede the activation of high-level system functions.
- Critical Performance Applications: In scenarios where speed is crucial, such as real-time computing in aerospace applications, simulation, and gaming graphics, assembly can provide the performance edge. By optimizing critical sections of code, assembly ensures minimal delay and maximum efficiency.
- Security and Reverse Engineering: Assembly language is also pivotal in the fields of security, where understanding malware at a low level can be crucial for defense mechanisms, and in reverse engineering, where it is used to dissect legacy code or binary executables without source code.
- Educational Purposes: Learning assembly language is valuable for students and professionals looking to gain a deeper understanding of how software interacts with hardware. This knowledge is crucial for optimizing software, troubleshooting complex issues, and developing a comprehensive skill set in computer science.
These applications illustrate why assembly language, despite its challenges and the dominance of high-level languages, remains a vital skill and tool in the arsenal of modern programmers and engineers. As we explore the initial steps to learning assembly in the next chapter, keep in mind these practical applications that could enhance your programming expertise and career opportunities.
4. Benefits of Knowing Assembly Language
Learning assembly language offers several unique advantages, particularly for those aiming to deepen their technical expertise:
- Performance Optimization: Understanding assembly allows programmers to optimize critical sections of code that need to run at maximum efficiency. This is especially useful in performance-sensitive applications like video games or real-time systems.
- Greater Control Over Hardware: Assembly provides direct access to hardware resources, offering a level of control not possible with high-level languages. This capability is crucial in fields like embedded systems development where hardware interaction is frequent.
- Enhanced Debugging Skills: Knowledge of assembly can significantly improve debugging skills. Seeing how high-level constructs break down into assembly code helps identify and fix performance bottlenecks and subtle bugs more effectively.
- Better Understanding of Underlying Mechanisms: Learning assembly can lead to a deeper understanding of how software interacts with hardware, how operating systems work, and how applications execute at the machine level, providing a comprehensive view of internal computer operations.
- Career Advancement: Proficiency in assembly language can be a valuable asset in areas such as systems programming, embedded systems development, and security analysis, potentially opening up new career opportunities.
Through these benefits, knowledge of assembly language not only enhances technical proficiency but also empowers programmers with the tools and insights needed to excel in the evolving landscape of computing. As we continue to explore the common challenges faced in assembly programming, remember that the skills developed through overcoming these challenges are invaluable in any programmer’s career.
5. Common Challenges in Assembly Programming
Learning and effectively using assembly language comes with several challenges, reflective of its detailed and intricate nature. Here are some common hurdles that programmers may encounter:
- Complex Syntax: Assembly language has a steep learning curve due to its terse and mnemonic-based syntax. Unlike high-level languages that feature comprehensive syntax and structured commands, assembly requires precise commands that directly manipulate CPU functions and memory.
- Platform Dependency: Assembly code is inherently tied to a specific type of processor architecture. This means code written for one type of CPU (e.g., Intel x86) will not work on another (e.g., ARM), without significant modifications. This lack of portability can complicate development processes, especially in multi-platform environments.
- Debugging Difficulty: Debugging assembly can be particularly challenging as errors may result from subtle mismanagement of system resources like registers and memory addresses. The low-level nature of the language can make it difficult to trace and rectify bugs, as opposed to high-level languages where debugging tools and error messages provide more context.
- Limited Resources and Support: Given the niche application of assembly language, there are fewer learning resources, communities, and modern development tools compared to more popular languages. This can make self-learning and problem-solving more challenging for new programmers.
- Verbose Code for Simple Tasks: Tasks that are trivial to implement in high-level languages can require lengthy and complex assembly code. This can lead to longer development times and increased potential for errors.
Addressing these challenges requires patience, practice, and a solid understanding of both the hardware and software aspects of computing systems. Despite these difficulties, the benefits of mastering assembly language, as discussed earlier, can significantly outweigh the hurdles for many developers.
6. Future of Assembly Language
Despite the dominance of high-level programming languages, the future of assembly language remains secure due to its unique capabilities and roles. Here’s how assembly language is expected to continue playing a crucial part in the world of programming:
- Continued Use in Embedded Systems: As long as there are resource-constrained environments and systems requiring high efficiency, assembly language will be essential. Its ability to maximize hardware performance is unmatched by high-level languages.
- Importance in Cybersecurity: Assembly language skills are invaluable for cybersecurity professionals, particularly in areas like malware analysis and system security, where understanding the lowest levels of computer operations is crucial.
- Educational Value: Assembly language continues to be an important educational tool for computer science students, offering deep insights into the workings of modern computers and helping develop a thorough grounding in computational thinking.
- Niche Applications: In specialized fields that demand extreme performance optimization or direct hardware manipulation (e.g., certain types of game development, custom operating system development, real-time military applications), assembly remains indispensable.
In the future, while fewer programmers may need to use assembly regularly, those who do will find it a powerful tool that offers a level of control and efficiency unmatched by other languages.
7. References
The information in this article is supported by various authoritative sources that provide further reading on the subject of assembly language and its applications:
- “Programming from the Ground Up” by Jonathan Bartlett – An introduction to programming using Linux assembly language.
- “The Art of Assembly Language” by Randall Hyde – Comprehensive coverage of assembly language programming with a focus on practical techniques and applications.
- “Computer Organization and Design MIPS Edition” by David A. Patterson and John L. Hennessy – Provides insight into the relationship between software and hardware, relevant for understanding assembly language in the context of computer architecture.
- RFC 20: ASCII format for Network Interchange – While not directly about assembly language, understanding the ASCII standard is crucial for low-level programming tasks involving text processing.
- “Assembly Language for x86 Processors” by Kip Irvine – Focuses on assembly language programming with an x86 processor architecture, used widely in educational contexts.