Imagine if every musician in an orchestra decided to play their own version of Beethoven’s Symphony No. 9. The result would be chaotic, to say the least. This is exactly what happens in web development projects when developers do not follow a common set of coding standards. I invite you to explore the melodious world of coding standards.
In this article, we’ll dissect these standards piece by piece, not only to understand what they are but also to learn how to effectively teach them to the next generation of web maestros – our junior web developers.
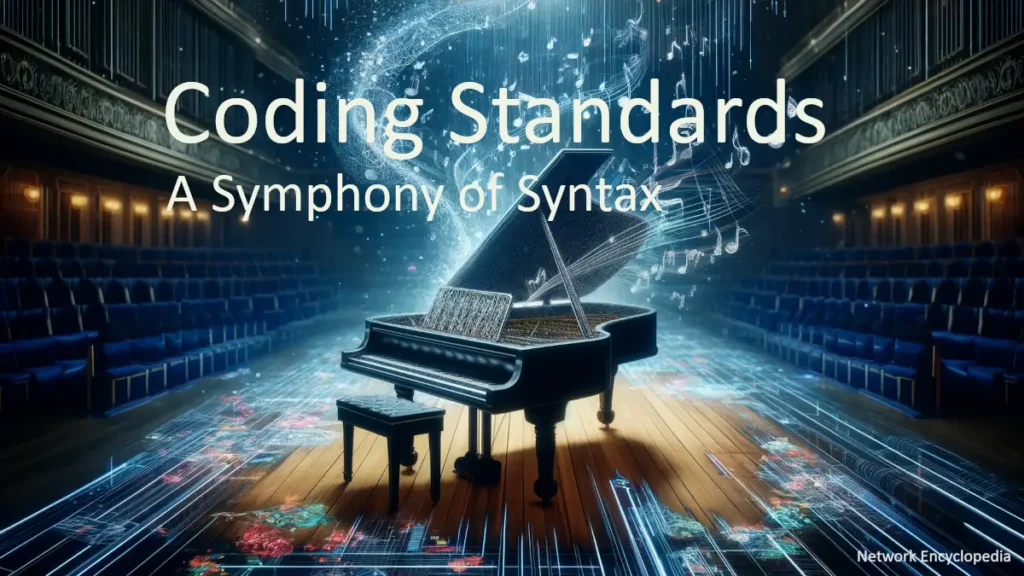
Chapters to Tune Into:
- Decoding the Standards: What Are Coding Standards?
- Conducting the Orchestra: Teaching Coding Standards Effectively
- Tools of the Trade: Linters, Formatters, and Other Aides
- Rehearsing the Code: Practice Techniques for Juniors
- Encore: Cultivating a Culture of Quality and Consistency
1. Decoding the Standards: What Are Coding Standards?
Just as every well-composed piece of music has a structure that musicians understand and follow, coding standards provide a framework that developers use to write their code. These standards are the sheet music of programming, guiding developers through the complex symphony of software development.
Coding standards are crucial for ensuring that a codebase is maintainable, scalable, and understandable. Here’s a more detailed look at the key areas of coding standards:
1.1. Naming Conventions
Naming conventions are critical because they help ensure that the code is intuitive and that its intent is immediately clear to any developer (or future you). Good naming conventions streamline the process of understanding the software, making it easier to maintain and modify. Here are some general principles:
- Descriptive Names: Use names that describe what the variable, function, class, or module does. For instance, a function name like calculateTotalWeight() is preferable to a vague name like doTheThing().
- Consistency: Stick to a particular pattern throughout your project. For example, if you’re using camelCase for variables, continue to use camelCase throughout your codebase.
- Avoid Abbreviations and Single Letter Names: Except for common practices (like i for loop indices), avoid abbreviations and single letters. Names like userList are better than uL.
- Context Matters: Choose names appropriate to the context and that provide clear information about their use.
To read next: Programming Languages 101.
1.2. Formatting Rules
Formatting involves the physical appearance of the code. While it may not affect the functionality, proper formatting makes the code more readable and organized. Key aspects include:
- Indentation and Spacing: Consistent use of indentation (spaces vs. tabs, the number of characters per indent) defines hierarchical relationships between lines of code.
- Braces Style: Whether you place braces on the same line as the function, class, or control statement, or on a new line, keep it consistent.
- Line Length: Avoid lines that are too long; they should be easy to read on a standard screen without scrolling horizontally.
- File Structure: Organize code files logically. Group similar functions together and separate distinct components appropriately.
1.3. Commenting Practices
Comments in the code should help explain the “why” behind the “what.” Comments are not a substitute for poor naming but are there to provide additional clarity and reasoning:
- Relevant Comments: Comments should add value, explaining why something is done a certain way if it’s not immediately obvious.
- Maintain Comments: Outdated comments are as harmful as outdated code. Ensure comments are updated alongside the code they describe.
- Avoid Over-Commenting: Don’t state the obvious; focus on the complexities and nuances that might not be immediately evident from the code alone.
1.4. Error Handling
Effective error handling is crucial for building reliable and robust applications. It involves anticipating and coding for possible errors that might occur during execution.
- Use Exceptions Rather Than Return Codes: Exceptions can’t be ignored easily and separate the error-handling code from the main logic.
- Provide Useful Error Messages: When throwing exceptions, provide messages that can help diagnose issues quickly.
- Consistency: Use a consistent strategy across the whole application for managing exceptions.
- Fail Fast: Where possible, make the code fail as soon as an error condition is detected. This simplifies debugging and often secures the system.
1.5. Architecture Guidelines
Architecture guidelines ensure that the code not only meets the current requirements but is also adaptable to changing needs without requiring a complete rewrite.
- Modularity: Design the system as a set of modular components, which can be developed, tested, reused, and updated independently.
- Layering: Use layers to separate concerns, such as separating the data access layer from the business logic layer and the presentation layer.
- Use Design Patterns Where Appropriate: Design patterns are tried and tested solutions to common problems. Using them can help avoid subtle issues that can cause major problems.
- Code Reusability: Aim for reusability through components and modules. This reduces duplication, which in turn reduces the potential for errors and maintenance overhead.
Adhering to these guidelines helps in creating a codebase that is efficient, understandable, and maintainable. Remember, the ultimate goal of coding standards is to enhance productivity and foster a code environment that allows teams to collaborate more effectively.
Also read: What is a Sandbox?
In the next chapter, we’ll explore how to conduct this orchestra, creating a symphony of coders who play in perfect harmony through the effective teaching of coding standards. Stay tuned, and remember, in coding as in music, practice doesn’t make perfect, perfect practice makes perfect!
2. Conducting the Orchestra: Teaching Coding Standards Effectively
As the conductor of an orchestra shapes the overall sound of the ensemble, so too must educators shape the coding habits of junior web developers. Teaching coding standards effectively isn’t just about dictating rules; it’s about engaging with your ensemble—your students—in a way that inspires them to embrace these practices as their own.
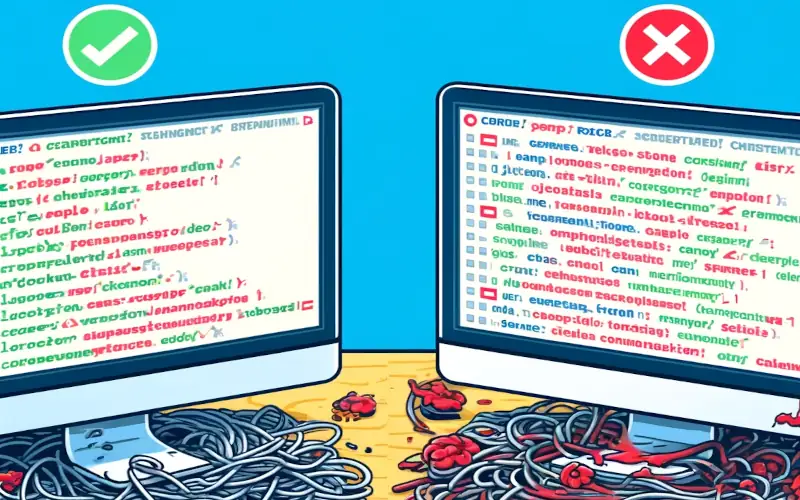
2.1. Start with the Why
Before diving into the hows, start with the whys. Explain the chaos of a codebase where everyone codes in their own style—akin to an orchestra where every tuba player decides on a different tune. Highlight how standards improve readability, reduce errors, and make maintenance easier, much like how sheet music keeps the orchestra in sync.
2.2. Interactive Examples
Use real-world scenarios to illustrate good and bad practices. Just as a side-by-side comparison of a symphony’s performance with and without a conductor can be enlightening, comparing well-structured code against a messy one can highlight the benefits of following standards.
2.3. Peer Reviews
Encourage code reviews among peers to foster a community learning environment. Like section rehearsals where musicians fine-tune their performance together, code reviews help developers learn from each other and enforce standards naturally.
2.4. Consistent Feedback
Offer continuous feedback, not unlike a conductor’s subtle cues during a performance. This can be through regular check-ins or automated feedback tools. The goal is to guide, not to scold.
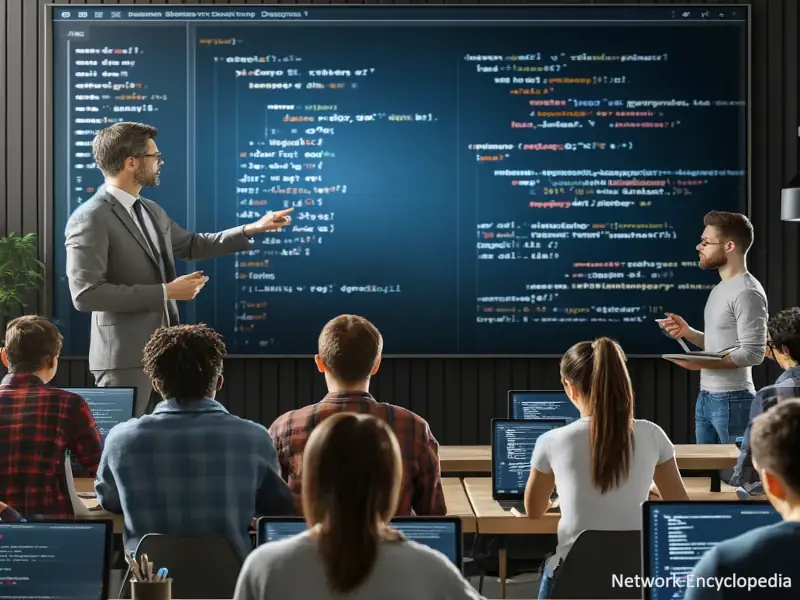
2.5. Gamify the Learning
Incorporate elements of gamification like badges or scores for adhering to standards. It’s like turning practice sessions into a playful competition, but instead of striving for the loudest note, the aim is for the cleanest code.
To read next: Software 2.0: The Evolution of Coding.
3. Tools of the Trade: Linters, Formatters, and Other Aides
Just as a musician relies on their instrument’s tuner or a metronome to ensure their performance is pitch-perfect, developers have their own tools to ensure their code is clean, efficient, and in tune with established standards.
3.1 Linters
Consider these the tuning forks of coding. Linters analyze code for errors and inconsistencies with the coding standards. They are like vigilant critics that never miss a note out of place. Popular linters include ESLint for JavaScript, which can be customized to match any coding standard your orchestra decides to follow.
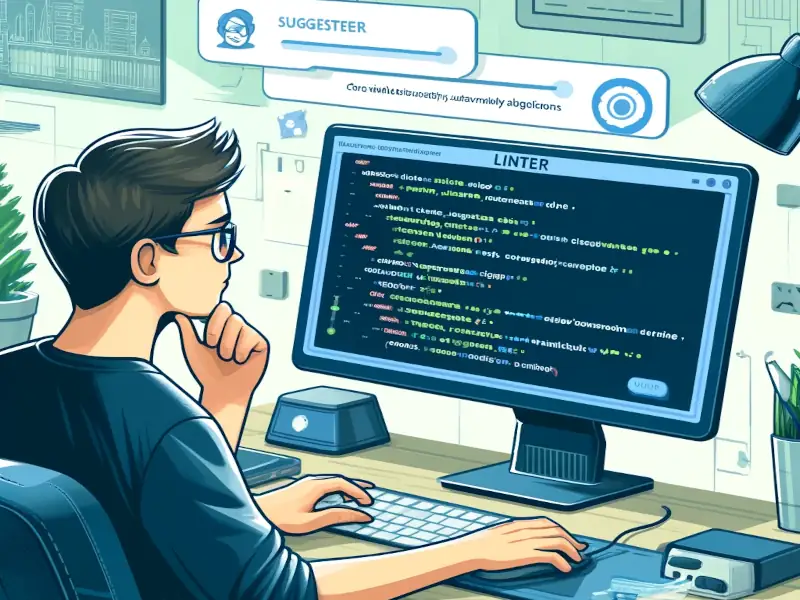
» See: What are Linters? [Codacy]
3.2 Formatters
If linters are the critics, formatters are the stylists. Tools like Prettier take your raw, unpolished code and reformat it into a stylistically consistent piece. It’s as if you handed your handwritten score to a copyist who returns a beautifully notated manuscript.
3.3. Integrated Development Environments (IDEs)
These are the full orchestral scores that contain all the parts for each instrument. IDEs like Visual Studio Code or JetBrains WebStorm come with built-in support for linters and formatters, and often provide real-time feedback and suggestions, much like a conductor providing real-time cues to an orchestra.
3.4. Version Control Systems
Think of these like rehearsal recordings. Tools like Git help manage changes in the codebase, allowing developers to revert to earlier versions if something goes awry, akin to a conductor reviewing a rehearsal tape and deciding to take a different approach to a particular passage.
3.5. Automated Code Review Tools
These can be likened to session recordings reviewed by an expert. Services like CodeClimate or SonarQube provide automated code reviews, highlighting potential issues and suggesting improvements based on predefined standards.
By leveraging these tools, we can ensure that every piece of code not only performs well but also plays beautifully in the grand symphony of a project. Remember, the goal is to make the code sing, and these tools are here to tune the voices.
To read next: Python Tic-Tac-Toe Game.
4. Rehearsing the Code: Practice Techniques for Juniors
Every musician knows that the key to a flawless performance is relentless practice. Similarly, junior web developers must hone their coding skills through constant practice, focused not just on solving problems, but on solving them right. Here’s how to make these practice sessions both beneficial and engaging:
4.1. Code Katas
Like scales in music practice, code katas are small, repeatable exercises that help developers refine their skills through repetition and variation. These exercises should focus on applying coding standards in a variety of scenarios, reinforcing the habits that make great code.
4.2. Pair Programming
Think of this as a duet, where two developers share a single workstation. One writes the code, while the other reviews each line as it is written. This not only improves code quality but also enhances learning, as the ‘observer’ can suggest adherence to coding standards in real-time.
4.3. Project-Based Learning
Assign small projects that require juniors to start from scratch, building their codebase with adherence to standards from the ground up. It’s like composing a short piece of music—they get to understand how each part fits into the larger whole.
4.4. Refactoring Sessions
Organize sessions where the sole focus is to refactor existing code to improve readability and efficiency while adhering to coding standards. This is akin to revising a piece to perfection, ensuring every note is in its right place.
4.5. Regular Quizzes
Implement short, frequent quizzes on coding standards. Like theory tests in music education, these help reinforce the knowledge and ensure juniors can recall and apply the standards when needed.
To read next: Start Your Python Journey: Project MiniPass.
5. Encore: Cultivating a Culture of Quality and Consistency
The grand finale in teaching coding standards is establishing a culture where quality and consistency are not just encouraged but celebrated. Here’s how to cultivate this environment:
5.1. Recognize and Reward
Just as standing ovations celebrate outstanding musical performances, recognize developers who consistently adhere to coding standards. Implement reward systems, like ‘Coder of the Month’, based on code quality metrics.
5.2. Consistent Messaging
From onboarding to daily stand-ups, emphasize the importance of coding standards. Just as motifs are repeated throughout a symphony to reinforce thematic elements, repeat your commitment to standards to embed them in your team’s psyche.
5.3. Mentorship Programs
Pair junior developers with seasoned mentors, much like apprentices with maestros. These relationships can provide ongoing support, guidance, and feedback, crucial for the development of a junior’s coding finesse.
5.5. Continuous Improvement
Encourage a mindset of continuous learning and improvement. Hold workshops, attend seminars, and review the latest coding standards and practices. Like musicians who continuously adapt to new music styles and techniques, developers should evolve with the changing tech landscape.
5.6. Open Discussions
Foster an environment where juniors feel comfortable discussing their code openly, whether it’s questions about best practices or seeking advice on handling specific challenges. Think of it as a group critique session, where everyone learns from each other’s compositions and critiques.
By embracing these practices, you not only teach coding standards effectively but also build a vibrant culture of excellence and harmony in your development team. Remember, the goal is not just to play the notes right but to make the music feel right—to make the code not just functional but exceptional.
Also read: Environment Variables: Unveiling the Hidden Power in Windows.